Building Real-time Chat Applications with Socket.io and Node.js
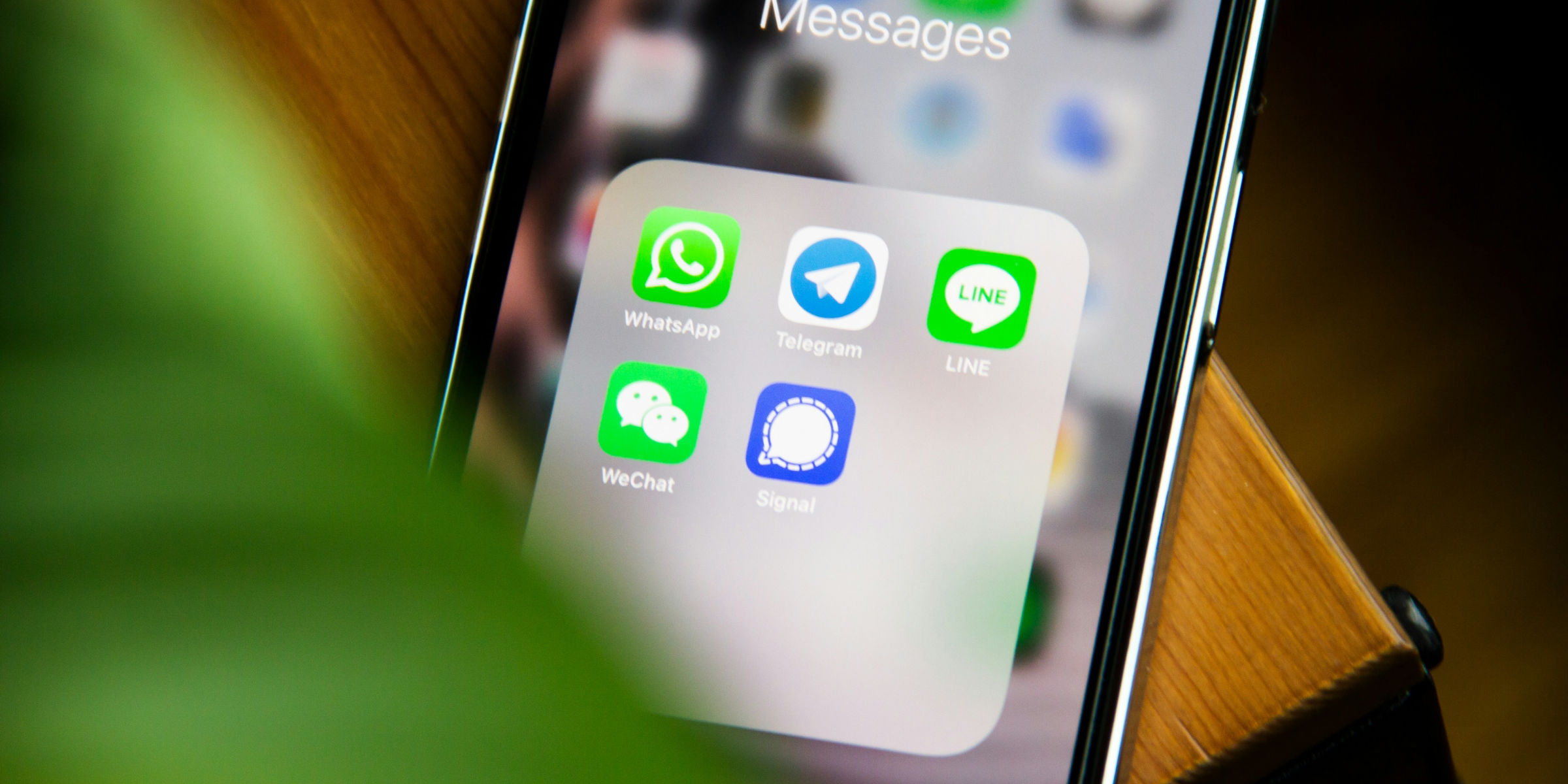
In this tutorial, we'll walk through the process of building a real-time chat application using Socket.io and Node.js. Real-time chat applications allow users to communicate instantly, making them ideal for messaging platforms, collaborative tools, and more. By the end of this guide, you'll have a basic understanding of how to set up a WebSocket server, handle events, and integrate the chat functionality with a front-end framework.
Prerequisites
Before we begin, make sure you have the following:
- Basic knowledge of JavaScript and Node.js.
- Node.js installed on your machine.
- A code editor such as Visual Studio Code or Sublime Text.
Setting Up the Project
Step 1: Initialize a new Node.js project
First, let's create a new directory for our project and initialize a new Node.js project using npm.
mkdir real-time-chat-app
cd real-time-chat-app
npm init -y
Step 2: Install dependencies
Next, install the necessary dependencies: Socket.io and Express.
npm install socket.io express
Creating the Server
Step 3: Setting up the WebSocket server
Now, let's create a server.js
file and set up our WebSocket server using Socket.io.
// server.js
const express = require('express');
const http = require('http');
const socketIo = require('socket.io');
const app = express();
const server = http.createServer(app);
const io = socketIo(server);
const PORT = process.env.PORT || 3000;
server.listen(PORT, () => {
console.log(`Server running on port ${PORT}`);
});
// Socket.io logic goes here
Handling Socket Events
Step 4: Handling connection and disconnection events
Socket.io provides built-in events for handling connection and disconnection of clients. Let's add logic to handle these events.
// server.js
io.on('connection', (socket) => {
console.log('A user connected');
socket.on('disconnect', () => {
console.log('User disconnected');
});
});
Implementing Chat Functionality
Step 5: Implementing chat messages
Now, let's implement the functionality to send and receive chat messages.
// server.js
io.on('connection', (socket) => {
console.log('A user connected');
socket.on('disconnect', () => {
console.log('User disconnected');
});
socket.on('chat message', (message) => {
console.log('message: ' + message);
io.emit('chat message', message);
});
});
Step 6: Integrating with the front-end
Finally, let's create a basic HTML file to serve as our front-end.
<!-- index.html -->
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Real-time Chat</title>
</head>
<body>
<ul id="messages"></ul>
<form id="form" action="">
<input id="input" autocomplete="off">
<button>Send</button>
</form>
<script src="/socket.io/socket.io.js"></script>
<script>
var socket = io();
var form = document.getElementById('form');
var input = document.getElementById('input');
form.addEventListener('submit', function(e) {
e.preventDefault();
if (input.value) {
socket.emit('chat message', input.value);
input.value = '';
}
});
socket.on('chat message', function(msg) {
var item = document.createElement('li');
item.textContent = msg;
document.getElementById('messages').appendChild(item);
});
</script>
</body>
</html>
Running the Application
Step 7: Run the server
Run the server using the following command:
node server.js
Open your web browser and navigate to http://localhost:3000
. You should see the chat interface. Open multiple tabs or browsers to test real-time communication.
Conclusion
Congratulations! You've successfully built a real-time chat application using Socket.io and Node.js. You can further enhance this application by adding features like user authentication, private messaging, or message persistence. Experiment with different front-end frameworks and customize the design to fit your needs. Happy coding!
Consult us for free?
View More