Enhancing Code Reusability with the Factory Pattern
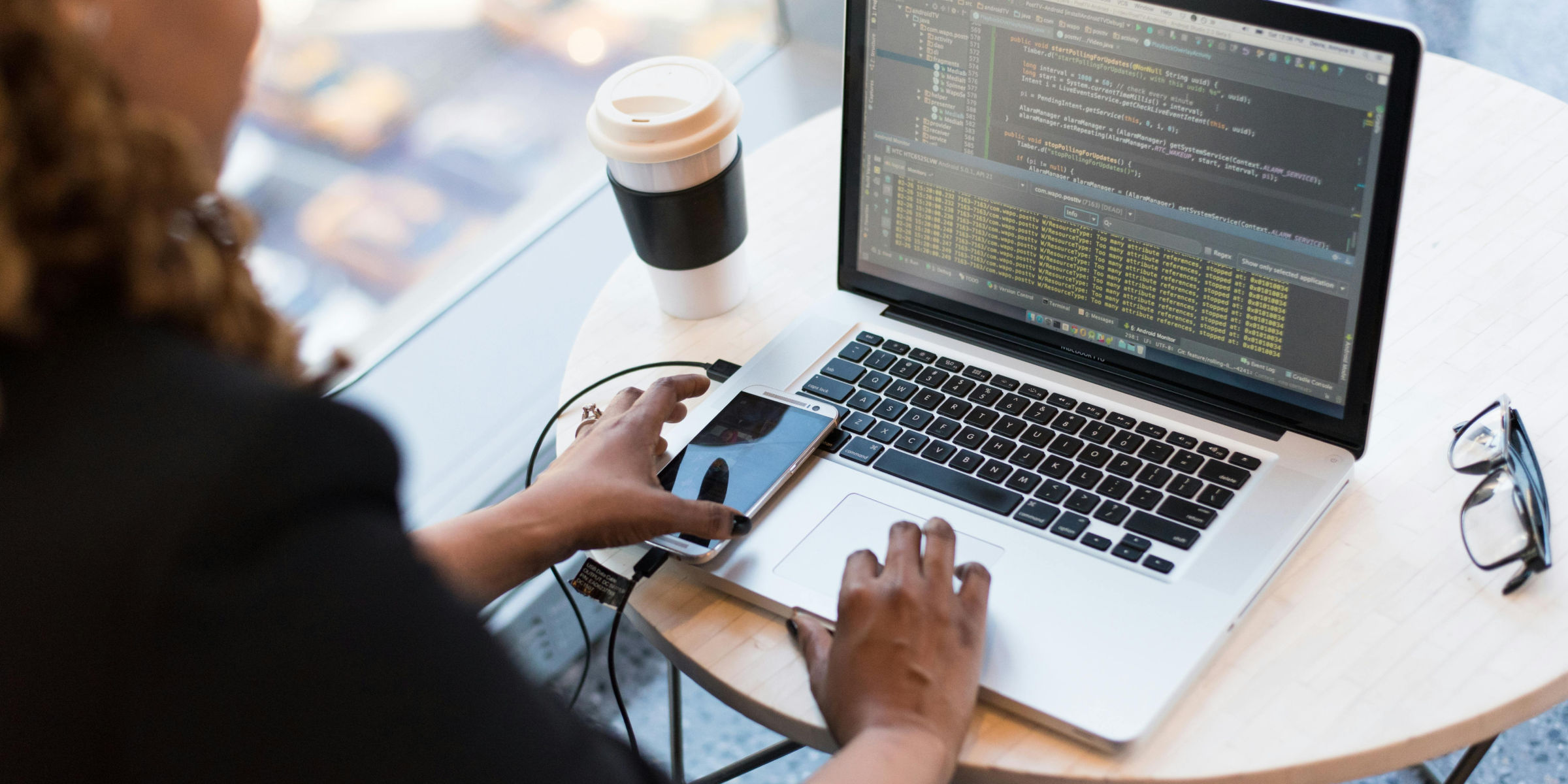
In modern JavaScript development, maintaining clean, reusable code is paramount. One design pattern that greatly aids in achieving this goal is the Factory pattern. By encapsulating object creation logic, the Factory pattern promotes code reusability and simplifies the process of creating complex objects. In this blog post, we'll explore the Factory pattern and its variations in JavaScript, including Factory Functions and Abstract Factories, and discuss how they enhance code reusability.
Understanding the Factory Pattern
At its core, the Factory pattern is a creational design pattern that provides an interface for creating objects without specifying their concrete classes. Instead of directly invoking a constructor to create objects, clients use a factory method or function to create instances based on certain criteria.
Key Components of the Factory Pattern
- Factory: The central component responsible for creating objects based on given parameters or conditions.
- Product: The objects created by the factory. These can be of various types, but they typically share a common interface or base class.
Implementing Factory Functions in JavaScript
In JavaScript, Factory Functions are a common implementation of the Factory pattern. These functions return new instances of objects based on provided parameters or configurations.
Example of a Fact
function createCar(type) {
switch (type) {
case 'sedan':
return new Sedan();
case 'suv':
return new SUV();
default:
throw new Error('Invalid car type');
}
}
const myCar = createCar('sedan');
In this example, the createCar
function acts as a factory, generating instances of different types of cars based on the provided type
parameter.
Advantages of Factory Functions
- Encapsulation: Object creation logic is encapsulated within the factory function, promoting modularity and separation of concerns.
- Abstraction: Clients interact with the factory function without needing to know the specifics of object creation.
- Flexibility: Easily extendable to accommodate new types of objects without modifying existing code.
Abstract Factory Pattern
While Factory Functions are suitable for creating individual objects, the Abstract Factory pattern takes it a step further by providing an interface for creating families of related or dependent objects. This pattern is particularly useful when dealing with complex systems composed of multiple interrelated components.
Example of an Abstract Factory
class CarFactory {
createEngine() {
throw new Error('Method not implemented');
}
createTire() {
throw new Error('Method not implemented');
}
}
class SedanFactory extends CarFactory {
createEngine() {
return new SedanEngine();
}
createTire() {
return new SedanTire();
}
}
class SUVFactory extends CarFactory {
createEngine() {
return new SUVEngine();
}
createTire() {
return new SUVTire();
}
}
const sedanFactory = new SedanFactory();
const suvFactory = new SUVFactory();
const sedanEngine = sedanFactory.createEngine();
const suvTire = suvFactory.createTire();
In this example, CarFactory
defines the interface for creating engines and tires, while SedanFactory
and SUVFactory
provide concrete implementations for creating sedan and SUV components, respectively.
Benefits of Abstract Factories
- Encapsulation of Related Object Creation: Abstract Factories encapsulate the creation of families of related objects, ensuring their compatibility and consistency.
- High Level of Abstraction: Clients interact with the Abstract Factory without needing to know the specific implementations of the created objects.
- Support for Variability: Abstract Factories allow for easy swapping of entire families of objects, promoting flexibility and maintainability.
Conclusion
The Factory pattern and its variations, such as Factory Functions and Abstract Factories, are powerful tools for enhancing code reusability and promoting clean, modular design in JavaScript applications. By encapsulating object creation logic and providing flexible interfaces for object instantiation, these patterns simplify complex systems and facilitate maintenance and scalability. Incorporating these patterns into your development workflow can lead to more robust and maintainable codebases.
Consult us for free?
View More