API Rate Limiting and Throttling with Express.js
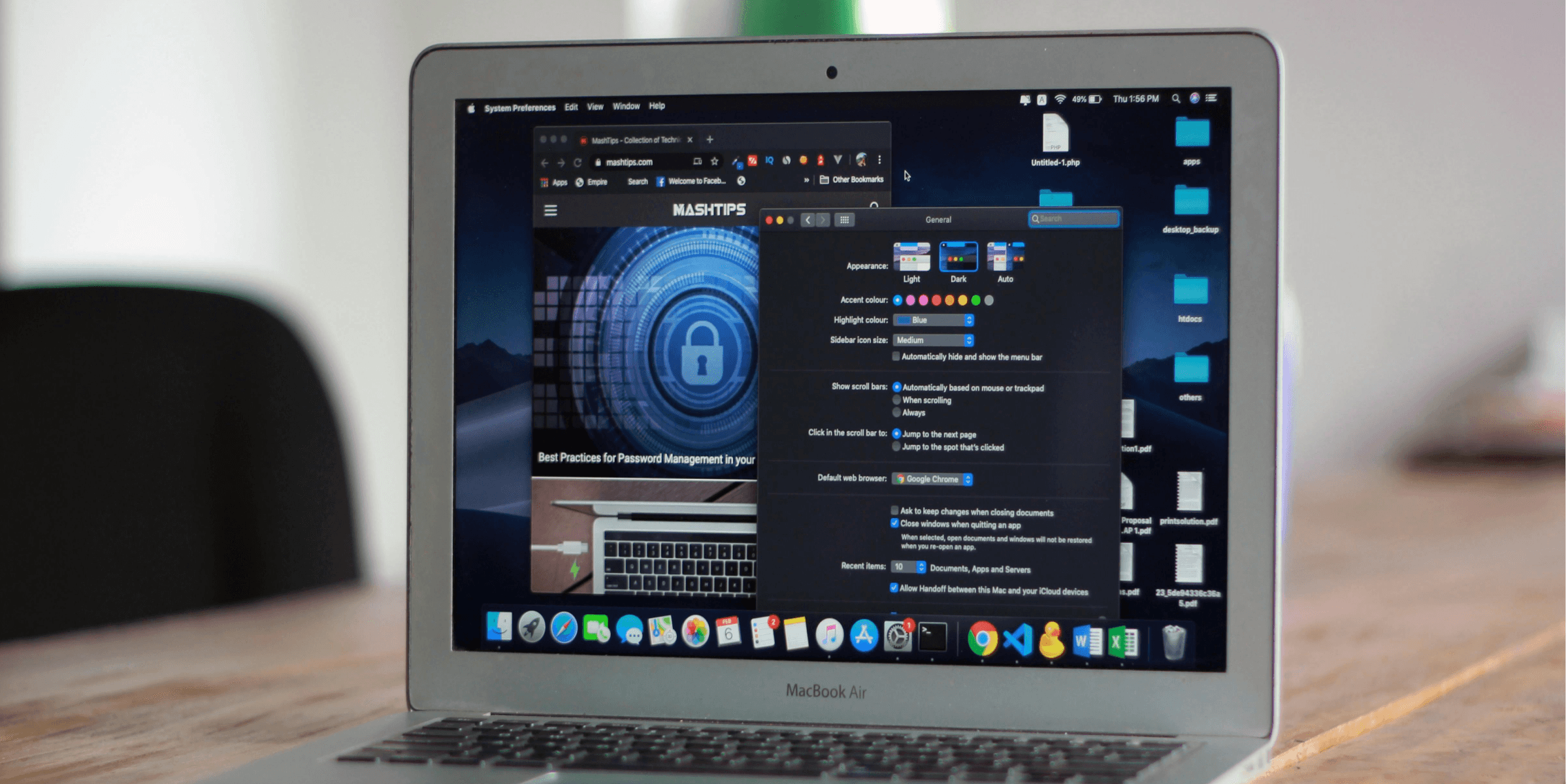
APIs are the backbone of modern web applications, enabling seamless communication between different services and clients. However, without proper controls, APIs can be vulnerable to abuse, such as DDoS attacks, excessive usage, or other forms of resource exploitation. Implementing rate limiting and throttling is essential to protect your API, ensure fair usage, and maintain performance and availability. In this blog, we’ll discuss the importance of rate limiting and throttling and how to implement them in an Express.js application using middleware like `express-rate-limit`.
Understanding Rate Limiting and Throttling
What is Rate Limiting?
Rate limiting is a technique used to control the number of requests a client can make to an API within a specified time period. This helps prevent abuse and ensures that resources are used efficiently. For example, you might limit a user to 100 requests per hour. If the user exceeds this limit, the API will reject further requests until the time period resets.
What is Throttling?
Throttling, often used interchangeably with rate limiting, generally refers to controlling the rate at which requests are processed to ensure the server remains responsive. It can involve delaying the processing of requests or reducing the priority of some requests to maintain overall system performance.
Importance of Rate Limiting and Throttling
1. Preventing Abuse and Misuse
Without rate limiting, malicious users could flood your API with requests, leading to service disruptions. Rate limiting ensures that no single user can monopolize your API resources.
2. Ensuring Fair Usage
APIs often serve multiple clients. Rate limiting ensures that resources are distributed fairly among all users, preventing scenarios where a few users consume the majority of resources.
3. Enhancing Security
Rate limiting can act as a deterrent against brute-force attacks, where an attacker tries numerous combinations to breach security measures. Limiting the number of attempts can significantly reduce the risk.
4. Maintaining Performance and Availability
By controlling the rate of incoming requests, rate limiting helps maintain the performance and availability of your API. This is particularly important in high-traffic scenarios where sudden spikes in requests could overwhelm the server.
5. Cost Management
For APIs that rely on third-party services with usage costs, rate limiting can help manage expenses by controlling the number of requests made to those services.
Implementing Rate Limiting in Express.js
Using Middleware for Rate Limiting
Express.js, being a flexible and minimalist web framework, allows for easy integration of middleware to implement rate limiting. One popular middleware for this purpose is `express-rate-limit`. This middleware provides a simple yet powerful way to control the rate of requests.
Steps to Implement Rate Limiting
1. Install the Middleware
- Add `express-rate-limit` to your project. This middleware handles the logic for rate limiting, allowing you to configure and apply limits easily.
2. Configure Rate Limiting
- Set up the rate limiting rules, such as the maximum number of requests allowed per time period and the response behavior when the limit is exceeded. You can customize these settings to match your application’s requirements.
3. Apply the Middleware
- Integrate the middleware into your Express.js application. This typically involves applying the middleware globally or to specific routes where rate limiting is needed. This ensures that all incoming requests are checked against the configured limits.
Best Practices for Rate Limiting
1. Define Clear Limits
- Set appropriate limits based on your API’s capacity and expected usage. Consider different limits for different types of users (e.g., free vs. premium).
2. Provide Clear Feedback
- When a request is rate-limited, provide clear feedback to the client, including information on when they can retry. This improves the user experience and helps clients understand the restrictions.
3. Monitor and Adjust
- Regularly monitor the effectiveness of your rate limiting rules. Use logs and analytics to understand usage patterns and adjust limits as needed to balance protection and usability.
4. Consider User Identity
- Implement rate limiting based on user identity (e.g., API keys or user accounts) rather than IP addresses, which can be shared by multiple users or changed frequently.
5. Combine with Other Security Measures
- Rate limiting should be part of a broader security strategy. Combine it with other measures such as authentication, authorization, and data validation to provide comprehensive protection.
Conclusion
Rate limiting and throttling are critical components for managing the load on your API, ensuring fair usage, and protecting against abuse. With Express.js and middleware like `express-rate-limit`, implementing these controls is straightforward and highly configurable. By adopting rate limiting, you can enhance the security, performance, and reliability of your API, providing a better experience for all users. If you haven’t already implemented rate limiting in your API, now is the time to do so and safeguard your services against potential threats and misuse.
Consult us for free?
View More