Advanced State Management Patterns in React
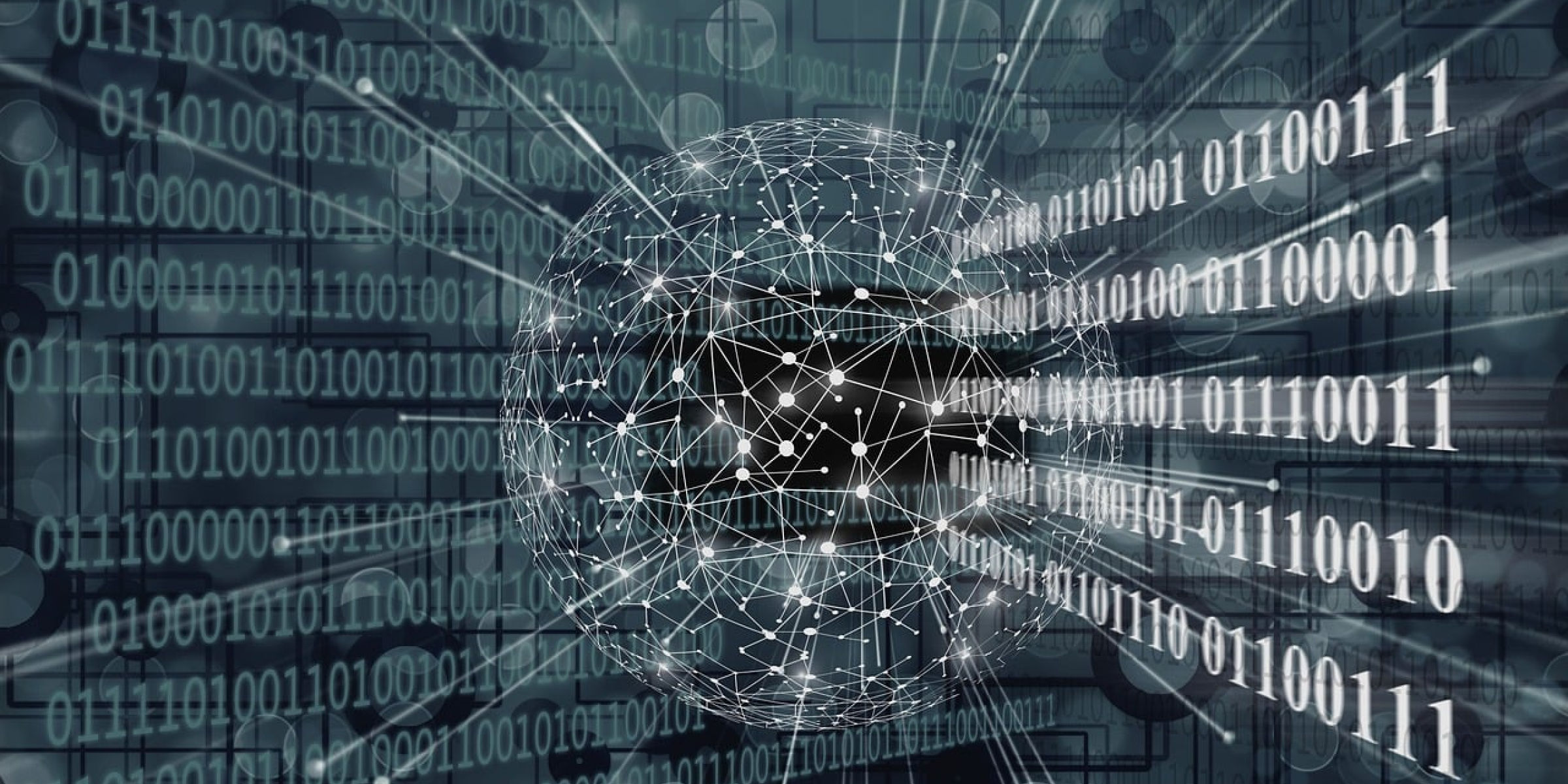
In React applications, managing state effectively is crucial for building scalable and maintainable user interfaces. While simple state management can be achieved with React's built-in state management, more complex applications may require advanced state management patterns. In this blog post, we'll explore three advanced state management patterns: the State Reducer pattern, the State Machine pattern, and Finite State Machines (FSM). We'll delve into their benefits, use cases, and how to implement them in React applications.
State Reducer Pattern
What is the State Reducer Pattern?
The State Reducer pattern involves encapsulating state logic within reducer functions. These reducers take the current state and an action as input and return the new state based on the action type.
Benefits of the State Reducer Pattern:
- Modularization: Reducers encapsulate state logic, promoting modularity and code organization.
- Predictable State Changes: By following a strict pattern, state changes become predictable and easier to reason about.
- Testability: Reducers can be easily unit tested, ensuring the correctness of state transitions.
Use Cases:
- Complex State Transitions: When state transitions involve complex logic or dependencies, the State Reducer pattern provides a structured approach.
- Shared State: When multiple components need to share and update the same state, reducers centralize the logic for managing that state.
State Machine Pattern
What is the State Machine Pattern?
The State Machine pattern models application behavior as a finite number of states and transitions between them. It's based on the principles of finite state machines (FSMs), where the application can only be in one state at a time, and transitions between states are triggered by events.
Benefits of the State Machine Pattern:
- Clarity and Understanding: State machines provide a clear visualization of application behavior, making it easier to understand and reason about complex state transitions.
- Prevent Invalid State Changes: By defining all possible states and transitions, state machines prevent invalid state changes, reducing bugs and edge cases.
- Scalability: As applications grow in complexity, state machines offer a scalable solution for managing state transitions.
Use Cases:
- User Interfaces with Complex Interactions: State machines are ideal for managing user interface behavior with complex interactions and multiple states.
- Workflow Management: Applications with defined workflows, such as order processing or form submissions, benefit from the clear structure provided by state machines.
Finite State Machines (FSM)
What are Finite State Machines (FSM)?
FSMs are mathematical models consisting of a finite number of states, transitions between states, and actions associated with transitions. In React applications, FSMs are used to model and manage complex state behavior.
Benefits of Finite State Machines (FSM):
- Formalization of State Logic: FSMs formalize state logic, providing a clear and structured approach to managing state transitions.
- Tooling Support: Various libraries and tools exist for working with FSMs, simplifying implementation and debugging.
- Reusability: FSMs can be reused across different parts of the application, promoting code reuse and maintainability.
Use Cases:
- Game Development: FSMs are commonly used in game development to model game states, player interactions, and AI behavior.
- Interactive User Interfaces: Applications with interactive user interfaces, such as wizards or multi-step processes, benefit from the clear structure provided by FSMs.
Conclusion
Advanced state management patterns such as the State Reducer pattern, State Machine pattern, and Finite State Machines (FSM) offer powerful tools for managing state in React applications. By adopting these patterns, developers can build more scalable, predictable, and maintainable user interfaces. Whether dealing with complex state transitions or interactive user interfaces, understanding and applying these patterns can greatly enhance the development process and improve the overall quality of React applications.
Consult us for free?
View More