Best Practices for Testing React Components
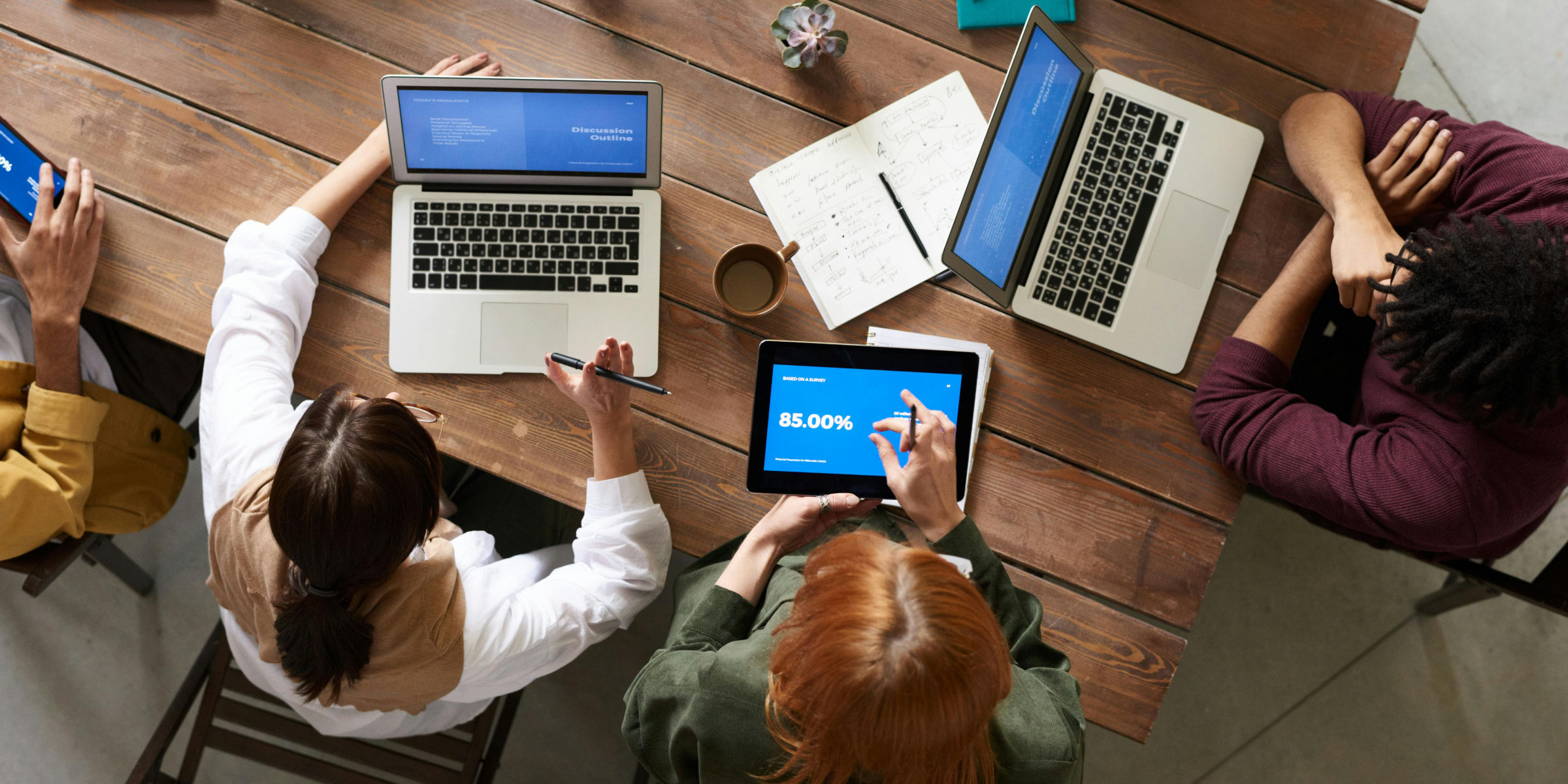
Setting Up Testing Environment
Choose a Testing Framework
Important Points:
- Select a testing framework such as Jest or Mocha, along with a testing library like React Testing Library or Enzyme.
- Jest is popular for its simplicity and built-in support for React, while React Testing Library encourages testing from a user's perspective.
Configuration
Important Points:
- Configure your testing environment to support JSX syntax and module imports using tools like Babel or TypeScript.
- Ensure that your configuration includes appropriate plugins for React-specific features like JSX and hooks.
Writing Tests
Focus on Behavior
Important Points:
- Write tests that focus on component behavior rather than implementation details.
- Test user interactions, state changes, and prop handling to ensure correct functionality.
Use Descriptive Test Names
Important Points:
- Use descriptive names for your tests to make them self-explanatory.
- Clear test names improve readability and help identify the purpose of each test case.
Test State Changes
Important Points:
- Test state changes triggered by user interactions or asynchronous operations.
- Ensure that the component updates its state correctly and reflects the changes in the UI.
Mock External Dependencies
Important Points:
- Use mocks to simulate external dependencies such as API calls or modules.
- Mocking allows isolated testing of components without relying on external services.
Test Edge Cases
Important Points:
- Consider edge cases and unexpected inputs when writing tests.
- Test boundary conditions, error handling, and corner cases to ensure robustness.
Test Coverage and Maintenance
Aim for High Test Coverage
Important Points:
- Strive for comprehensive test coverage to minimize the risk of regressions.
- Aim for a balance between unit tests, integration tests, and end-to-end tests to cover different aspects of your application.
Refactor and Update Tests Regularly
Important Points:
- Refactor tests as the codebase evolves to maintain readability and relevance.
- Update tests to accommodate changes in component behavior or requirements.
Continuous Integration
Important Points:
- Integrate testing into your continuous integration (CI) pipeline for automated testing.
- Use tools like Jenkins, Travis CI, or GitHub Actions to run tests automatically on every code push.
Conclusion
Testing React components is essential for ensuring the quality and reliability of your applications. By following best practices such as focusing on behavior, using descriptive test names, and aiming for high test coverage, you can build robust and maintainable React applications.
Implementing these practices not only improves the stability of your codebase but also enhances the confidence of developers and stakeholders in the application's functionality.
Consult us for free?
View More