Concurrency Design Patterns in Java: Thread Pool, Future, and Producer-Consumer
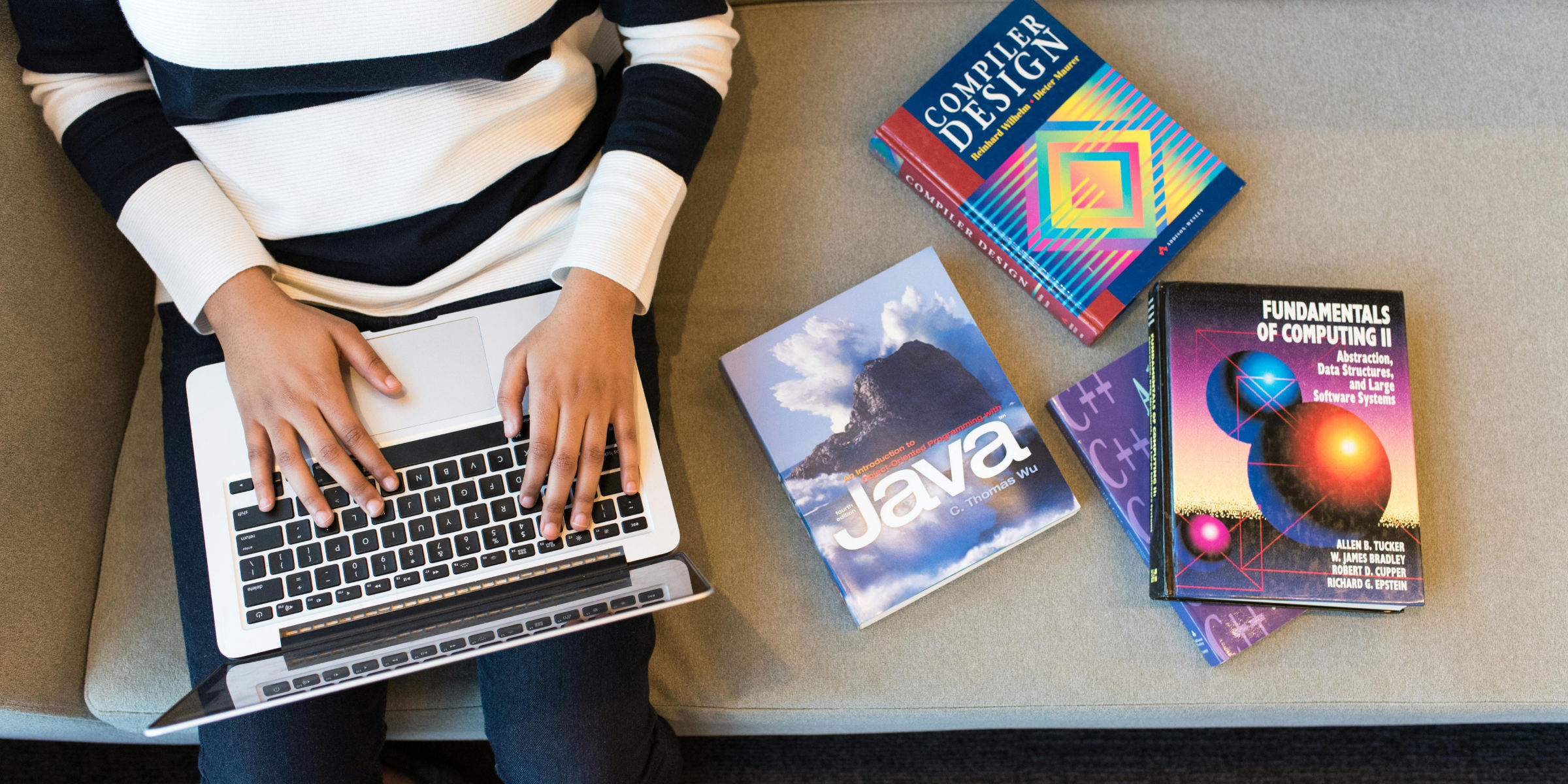
In Java, concurrency design patterns play a crucial role in building efficient and scalable applications that can handle multiple tasks simultaneously. In this blog post, we will delve into three important concurrency design patterns: Thread Pool, Future, and Producer-Consumer. These patterns provide solutions to common challenges encountered in concurrent programming, such as resource management, task execution, and synchronization.
Thread Pool Pattern
Introduction
A thread pool is a collection of pre-initialized threads that are ready to perform tasks. Instead of creating a new thread for each task, a thread pool reuses existing threads, reducing the overhead of thread creation and destruction.
Benefits
- Improved Performance: Thread pools reduce the overhead associated with thread creation, resulting in improved performance and responsiveness.
- Resource Management: By limiting the number of concurrent threads, thread pools prevent resource exhaustion and excessive context switching.
- Scalability: Thread pools allow you to control the number of threads based on system resources, making applications more scalable.
Implementation
- java.util.concurrent.ExecutorService: Java provides the ExecutorService interface to manage thread pools. You can create a thread pool using Executors factory methods, such as
newFixedThreadPool()
ornewCachedThreadPool()
. - ThreadPoolExecutor: For more advanced configurations, you can use ThreadPoolExecutor class directly, providing fine-grained control over thread pool parameters.
Best Practices
- Right-sizing: Choose an appropriate pool size based on the available resources and workload characteristics.
- Task Submission: Submit tasks to the thread pool using a suitable method like
submit()
orexecute()
. - Shutdown: Always shutdown the thread pool when it's no longer needed to release resources gracefully.
Future Pattern
Introduction
The Future pattern provides a way to represent the result of an asynchronous computation. It allows you to execute tasks asynchronously and retrieve their results later.
Benefits
- Asynchronous Execution: Futures enable asynchronous task execution, allowing the calling thread to perform other operations while waiting for the result.
- Result Retrieval: You can retrieve the result of a computation from the Future object when it becomes available.
- Exception Handling: Futures support exception handling, making it easier to propagate and handle errors in asynchronous tasks.
Implementation
- java.util.concurrent.Future: Java provides the Future interface to represent the result of an asynchronous computation.
- CompletableFuture: Introduced in Java 8, CompletableFuture extends the Future interface and provides additional functionalities like composition, chaining, and combining multiple asynchronous computations.
Best Practices
- Error Handling: Use methods like
get()
orjoin()
to retrieve the result, handling any exceptions that might occur during task execution. - Composition: Leverage CompletableFuture's methods like
thenApply()
,thenCompose()
, andthenCombine()
for composing asynchronous computations. - Timeouts: Consider specifying a timeout when retrieving the result from a Future to prevent indefinite waiting.
Producer-Consumer Pattern
Introduction
The Producer-Consumer pattern facilitates communication between two threads: one producing data (producer) and another consuming it (consumer). It helps in decoupling the production and consumption of data, ensuring thread safety and efficient resource utilization.
Benefits
- Decoupling: By separating the producer and consumer, the pattern allows them to operate independently, improving modularity and maintainability.
- Thread Safety: Proper synchronization mechanisms ensure that the producer and consumer threads can access shared data safely without race conditions.
- Efficient Resource Utilization: The pattern prevents resource wastage by regulating the flow of data between producers and consumers, avoiding overproduction or underconsumption.
Implementation
- BlockingQueue: Java provides BlockingQueue implementations such as ArrayBlockingQueue and LinkedBlockingQueue to implement the Producer-Consumer pattern efficiently.
- Synchronization: Use synchronization primitives like locks, conditions, or semaphores to coordinate access to shared data between producers and consumers.
Best Practices
- Buffer Size: Choose an appropriate buffer size for the BlockingQueue to balance between memory consumption and throughput.
- Error Handling: Implement proper error handling mechanisms to handle exceptions and edge cases gracefully.
- Shutdown: Ensure graceful shutdown of producer and consumer threads to avoid resource leaks and deadlocks.
Conclusion
Concurrency design patterns such as Thread Pool, Future, and Producer-Consumer are essential tools for building robust and efficient Java applications. By understanding these patterns and their implementations, developers can write concurrent code that is scalable, maintainable, and reliable. Whether you're developing multi-threaded applications or leveraging asynchronous processing, these patterns provide valuable techniques for effective concurrent programming in Java.
Consult us for free?
View More