Design Patterns and the Open-Closed Principle
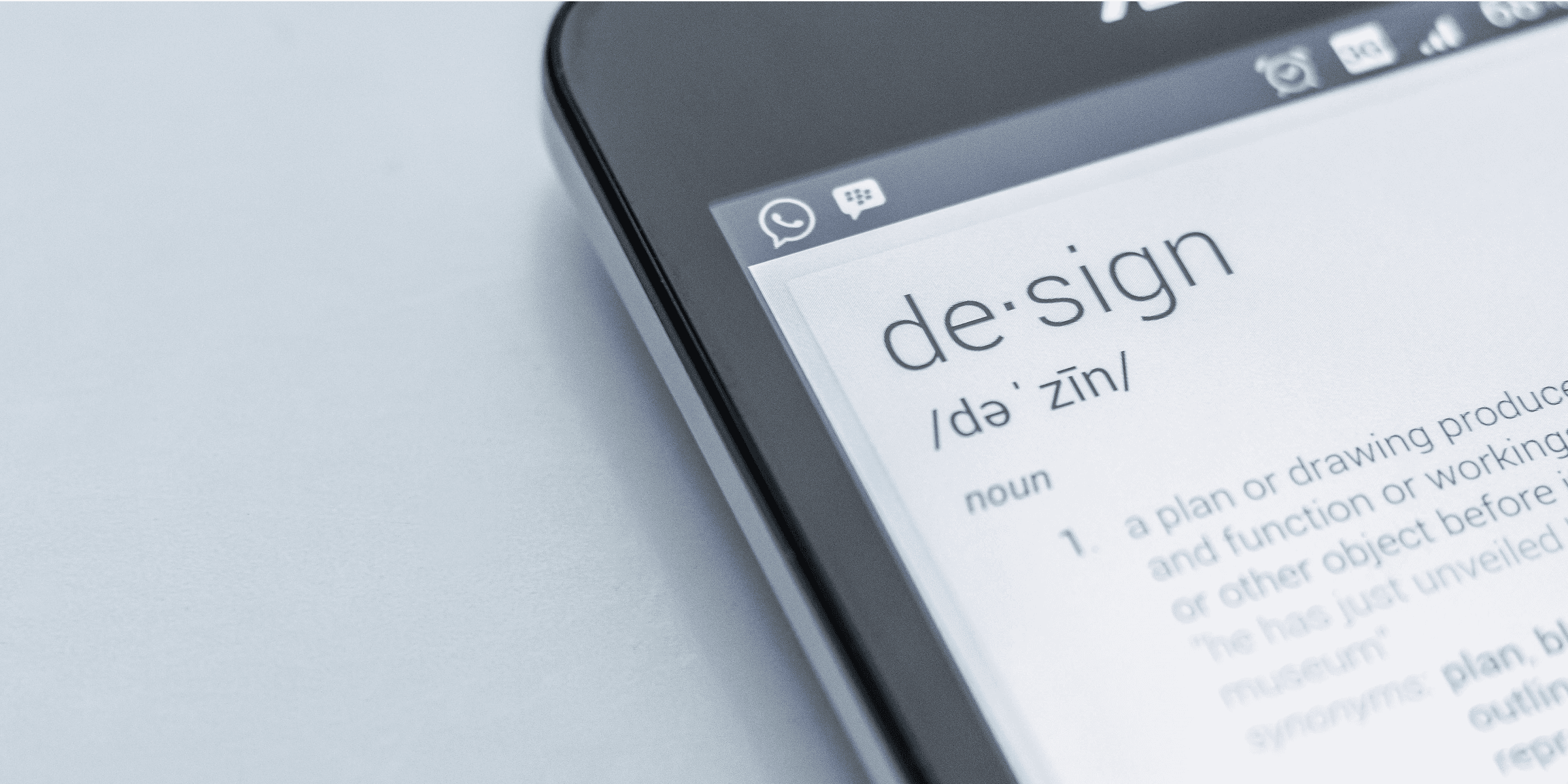
The Open-Closed Principle (OCP) is a fundamental principle in software engineering, advocating for classes to be open for extension but closed for modification. This principle fosters code stability, scalability, and maintainability by encouraging developers to build systems that can accommodate new functionality without altering existing code. Design patterns, as reusable solutions to common problems in software design, often embody the Open-Closed Principle in various ways. In this blog, we'll explore how design patterns such as the Strategy Pattern, Template Method Pattern, and Decorator Pattern adhere to and leverage the Open-Closed Principle.
Understanding the Open-Closed Principle
Before delving into design patterns, let's establish a clear understanding of the Open-Closed Principle. At its core, the OCP suggests that classes should be designed in a way that allows for extension through inheritance or composition, without requiring modification of their internal implementation. This ensures that existing code remains unchanged while new features can be added through extensions or variations.
Strategy Pattern
The Strategy Pattern is a behavioral design pattern that enables the definition of a family of algorithms, encapsulating each one, and making them interchangeable. It allows the algorithm to vary independently from clients that use it, promoting code reuse and flexibility. In terms of the Open-Closed Principle:
Open for Extension
- New strategies can be added without altering the existing codebase.
- Clients can easily incorporate new behaviors by implementing the strategy interface.
Closed for Modification
- Once a strategy interface is defined, it remains stable and doesn't require modification.
- Changes in behavior are achieved through composition, not by altering existing code.
Template Method Pattern
The Template Method Pattern is a behavioral design pattern that defines the skeleton of an algorithm in the superclass but allows subclasses to override specific steps of the algorithm without changing its structure. This pattern promotes code reuse and provides a framework for defining algorithms with varying implementations. Regarding the Open-Closed Principle:
Open for Extension
- Subclasses can extend and customize specific parts of the algorithm by overriding template methods.
- New variations of the algorithm can be introduced without modifying the existing codebase.
Closed for Modification
- The structure of the algorithm defined in the superclass remains unchanged.
- Subclasses can only extend or override specific parts of the algorithm, preserving the integrity of the original implementation.
Decorator Pattern
The Decorator Pattern is a structural design pattern that allows behavior to be added to individual objects dynamically, without affecting the behavior of other objects from the same class. It provides an alternative to subclassing for extending functionality. In terms of the Open-Closed Principle:
Open for Extension
- Additional behaviors can be added to objects by wrapping them with decorators.
- New decorators can be created to introduce new features or modify existing ones.
Closed for Modification
- The core functionality of objects remains unchanged.
- Decorators adhere to a common interface, allowing them to be combined and composed flexibly without modifying existing code.
Conclusion
Design patterns serve as powerful tools for implementing software solutions that adhere to fundamental principles such as the Open-Closed Principle. By leveraging patterns like the Strategy Pattern, Template Method Pattern, and Decorator Pattern, developers can build systems that are open for extension but closed for modification, promoting code maintainability, flexibility, and scalability. Understanding these patterns and their alignment with core principles is essential for crafting robust and adaptable software architectures.
Consult us for free?
View More