Design Patterns for Python Microservices: Building Resilient and Scalable Systems
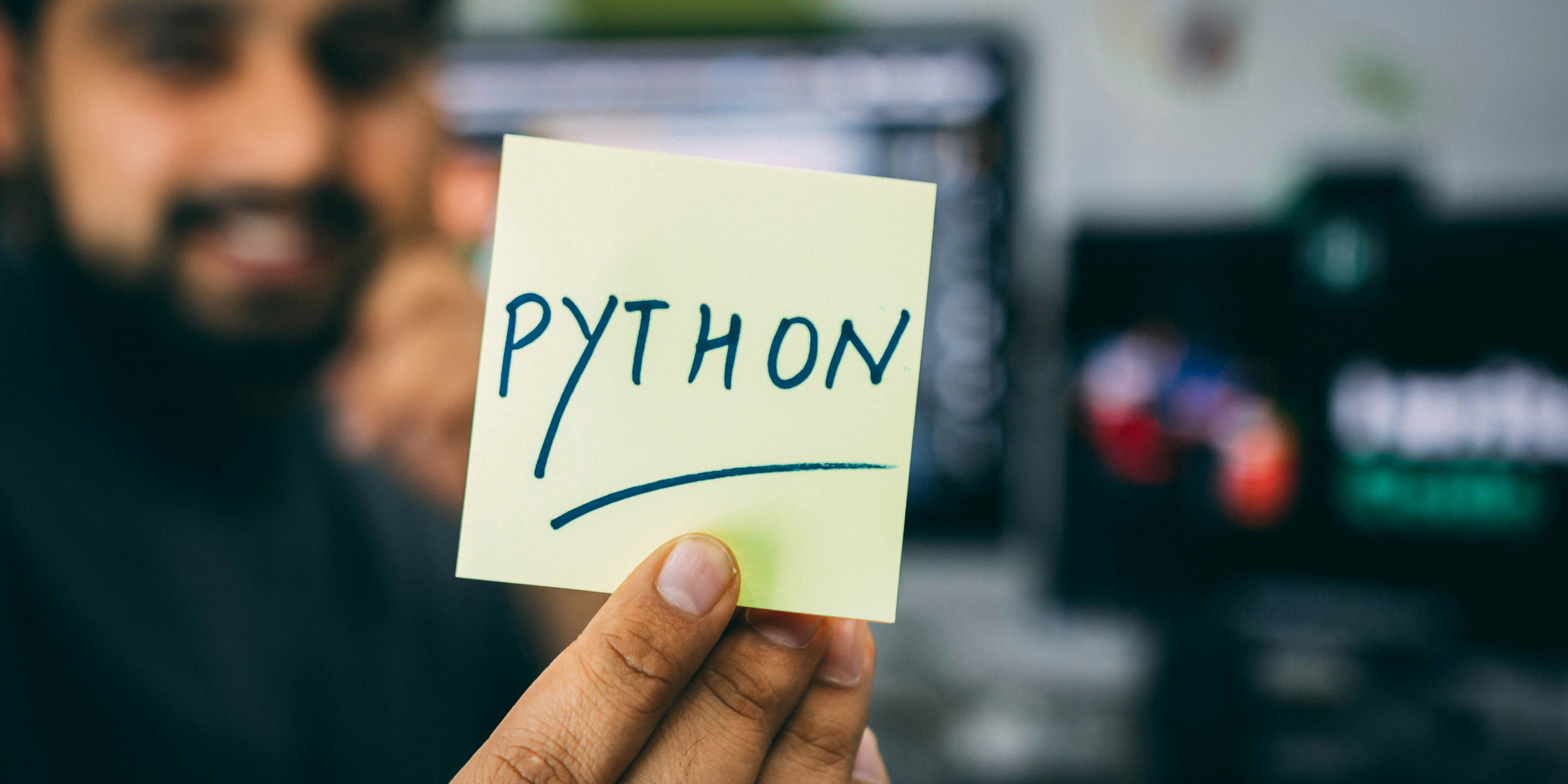
In the realm of modern software architecture, microservices have emerged as a popular approach for building complex applications. They offer benefits such as improved scalability, flexibility, and resilience. However, developing and maintaining a microservices architecture comes with its own set of challenges. To overcome these challenges and build robust systems, it's essential to employ appropriate design patterns. In this blog post, we'll delve into several design patterns suitable for developing microservices in Python, including Circuit Breaker, Saga, and CQRS (Command Query Responsibility Segregation). We'll explore how these patterns contribute to building resilient and scalable microservices architectures.
Introduction: Microservices Architecture in Python
Microservices architecture involves breaking down a monolithic application into smaller, independent services that can be developed, deployed, and scaled independently. Python, with its simplicity, flexibility, and rich ecosystem of libraries and frameworks, is well-suited for building microservices-based applications. However, as the number of services grows and the complexity increases, maintaining the reliability and scalability of the system becomes challenging. This is where design patterns play a crucial role.
1. Circuit Breaker Pattern
The Circuit Breaker pattern is a design pattern used to handle faults and failures in distributed systems. It prevents a service from continuously making requests to another service that is experiencing issues, thus preventing cascading failures. In Python, libraries like hystrix-python
provide implementations of the Circuit Breaker pattern.
Example:
from hystrix import fallback, command
@fallback(fallback_method=falling_back)
@command
def make_request():
# Make HTTP request to another service
response = requests.get('http://example.com')
return response.json()
def falling_back():
# Fallback logic when the circuit is open
return {"message": "Fallback response"}
2. Saga Pattern
The Saga pattern is used to maintain data consistency in a distributed transaction across multiple services. It decomposes a long-running transaction into a series of smaller, independent transactions that can be individually committed or rolled back. In Python, frameworks like saga-python
provide support for implementing Sagas.
Example:
from saga import Saga, saga_step
class OrderSaga(Saga):
@saga_step
def reserve_inventory(self, order_id):
# Reserve inventory for the order
pass
@saga_step
def charge_customer(self, order_id):
# Charge the customer for the order
pass
@saga_step
def ship_order(self, order_id):
# Ship the order to the customer
pass
@saga_step
def complete_order(self, order_id):
# Complete the order
pass
3. CQRS Pattern
The Command Query Responsibility Segregation (CQRS) pattern separates read and write operations for a data store. It allows for different models to be used for reading and writing data, optimizing performance and scalability. In Python, libraries like cqrs-python
provide utilities for implementing CQRS.
Example:
class CommandHandler:
def handle(self, command):
# Handle write operations
pass
class QueryHandler:
def handle(self, query):
# Handle read operations
pass
Conclusion
Design patterns play a crucial role in building resilient and scalable microservices architectures in Python. By leveraging patterns like Circuit Breaker, Saga, and CQRS, developers can effectively handle faults, maintain data consistency, and optimize performance. These patterns contribute to the overall robustness and reliability of microservices-based systems, enabling organizations to deliver high-quality software that meets the demands of modern, distributed applications.
Consult us for free?
View More