Behavioral Design Patterns for Node.js: Observer, Strategy, and Command Patterns
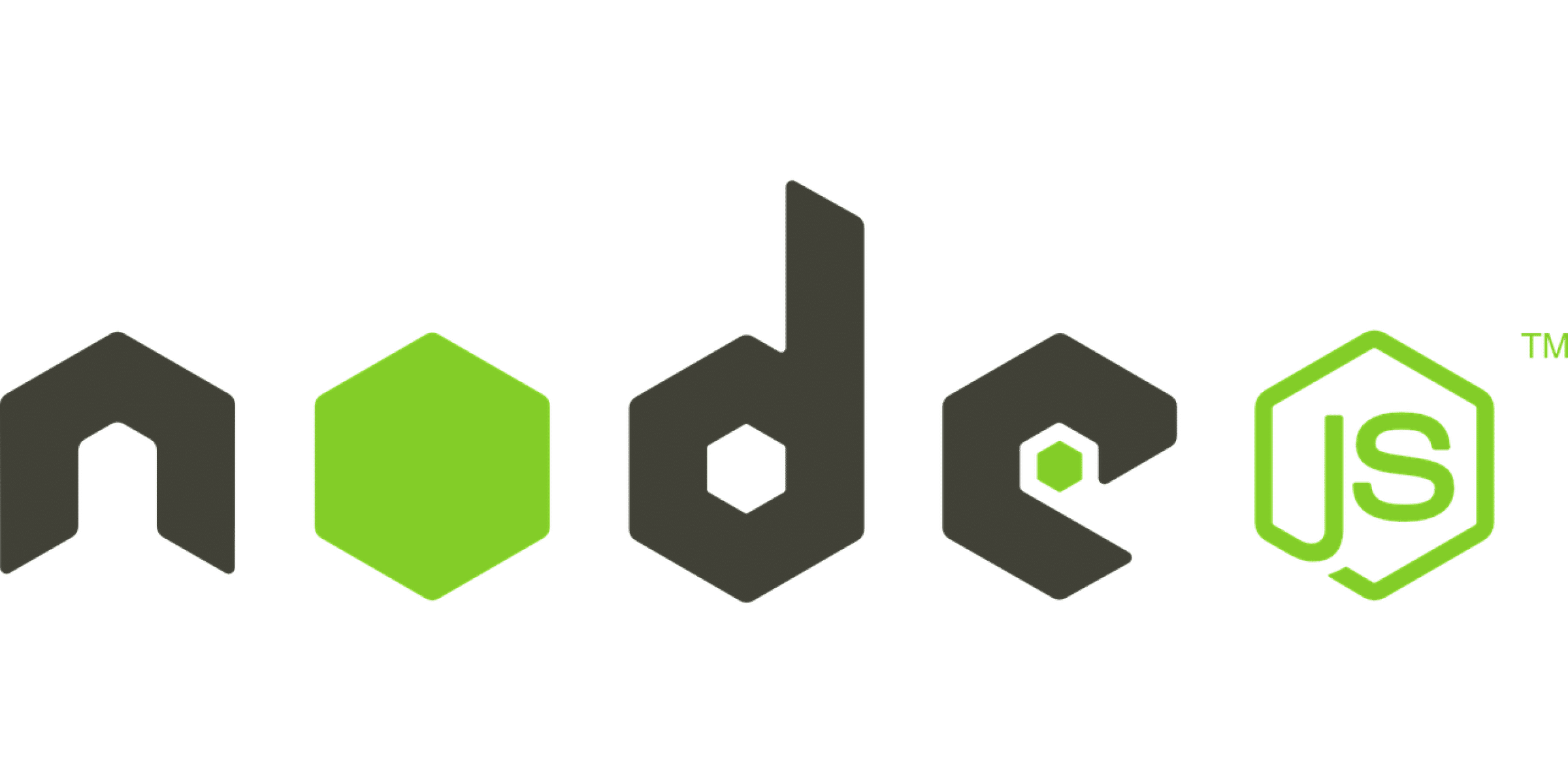
In software engineering, design patterns provide reusable solutions to common problems faced during software development. Behavioral design patterns, in particular, focus on how objects interact and communicate with each other. In this blog post, we'll delve into three key behavioral design patterns—Observer, Strategy, and Command Patterns—and explore how they can be implemented in Node.js applications.
What are Behavioral Design Patterns?
Behavioral design patterns are concerned with the interaction and communication between objects. They focus on how objects collaborate and delegate responsibilities to achieve specific behaviors within an application. These patterns promote loose coupling and flexibility in software design, making it easier to maintain and extend codebases over time.
Observer Pattern
The Observer Pattern is a behavioral design pattern where an object, known as the subject, maintains a list of its dependents, called observers, and notifies them of any state changes, typically by calling one of their methods. This pattern is widely used in event-driven architectures.
Key Components
- Subject: The object being observed. It maintains a list of observers and notifies them of state changes.
- Observer: The objects that are interested in the state changes of the subject. They register themselves with the subject and receive notifications.
Implementation in Node.js
// Subject
class Subject {
constructor() {
this.observers = [];
}
addObserver(observer) {
this.observers.push(observer);
}
notifyObservers(data) {
this.observers.forEach(observer => observer.update(data));
}
}
// Observer
class Observer {
update(data) {
console.log('Received data:', data);
}
}
// Usage
const subject = new Subject();
const observer1 = new Observer();
const observer2 = new Observer();
subject.addObserver(observer1);
subject.addObserver(observer2);
subject.notifyObservers('New data');
Important Points
- Loose Coupling: The Observer Pattern promotes loose coupling between subjects and observers, allowing for easier maintenance and scalability.
- Event-Driven Architecture: It is commonly used in event-driven architectures, such as in Node.js applications handling asynchronous operations.
Strategy Pattern
The Strategy Pattern is a behavioral design pattern that enables an algorithm's behavior to be selected at runtime. It defines a family of algorithms, encapsulates each one, and makes them interchangeable. This pattern allows the algorithm to vary independently from the clients that use it.
Key Components
- Context: The object that maintains a reference to the strategy object and delegates its behavior.
- Strategy: The interface or abstract class defining a family of algorithms.
- Concrete Strategies: The concrete implementations of the strategy interface.
Implementation in Node.js
// Strategy Interface
class SortingStrategy {
sort(array) {}
}
// Concrete Strategies
class BubbleSort extends SortingStrategy {
sort(array) {
return array.sort((a, b) => a - b);
}
}
class QuickSort extends SortingStrategy {
sort(array) {
return array.sort((a, b) => a - b);
}
}
// Context
class SortContext {
constructor(strategy) {
this.strategy = strategy;
}
setStrategy(strategy) {
this.strategy = strategy;
}
executeStrategy(array) {
return this.strategy.sort(array);
}
}
// Usage
const bubbleSortStrategy = new BubbleSort();
const sortContext = new SortContext(bubbleSortStrategy);
const array = [3, 1, 2];
console.log(sortContext.executeStrategy(array)); // [1, 2, 3]
const quickSortStrategy = new QuickSort();
sortContext.setStrategy(quickSortStrategy);
console.log(sortContext.executeStrategy(array)); // [1, 2, 3]
Important Points
- Encapsulation: The Strategy Pattern encapsulates algorithms, making them interchangeable without affecting the client's code.
- Run-Time Selection: It allows algorithms to be selected at runtime based on specific requirements or conditions.
Command Pattern
The Command Pattern is a behavioral design pattern that encapsulates a request as an object, thereby allowing for parameterization of clients with queues, requests, and operations. It decouples the sender from the receiver, providing flexibility in handling commands and allowing for the execution of operations at different times.
Key Components
- Command: The interface or abstract class defining the command's execution method.
- Concrete Command: The concrete implementations of the command interface, encapsulating a specific action.
- Invoker: The object that invokes the command and forwards the request to the appropriate receiver.
- Receiver: The object that performs the actual action associated with the command.
Implementation in Node.js
// Command Interface
class Command {
execute() {}
}
// Concrete Command
class LightOnCommand extends Command {
constructor(light) {
super();
this.light = light;
}
execute() {
this.light.turnOn();
}
}
// Receiver
class Light {
turnOn() {
console.log('Light is on');
}
}
// Invoker
class RemoteControl {
constructor() {
this.command = null;
}
setCommand(command) {
this.command = command;
}
pressButton() {
this.command.execute();
}
}
// Usage
const light = new Light();
const lightOnCommand = new LightOnCommand(light);
const remoteControl = new RemoteControl();
remoteControl.setCommand(lightOnCommand);
remoteControl.pressButton(); // Output: Light is on
Important Points
- Decoupling: The Command Pattern decouples the sender from the receiver, allowing for flexible and extensible code.
- Undo Operations: It enables undo operations by storing the history of commands executed, facilitating the reversal of actions if necessary.
Conclusion
In this blog post, we explored three important behavioral design patterns—Observer, Strategy, and Command Patterns—and their implementation in Node.js applications. By understanding these patterns and their use cases, developers can enhance the flexibility, scalability, and maintainability of their codebases. Whether it's handling asynchronous events, selecting algorithms at runtime, or encapsulating commands, behavioral design patterns provide valuable solutions to common software design challenges.
By incorporating Observer, Strategy, and Command Patterns into your Node.js applications, you can enhance flexibility and maintainability, ensuring that your codebase remains robust and scalable. These patterns offer valuable solutions to common software design challenges, empowering developers to build high-quality, modular applications.
Consult us for free?
View More