Managing Asynchronous Operations with the Promise and Async/Await Patterns in React
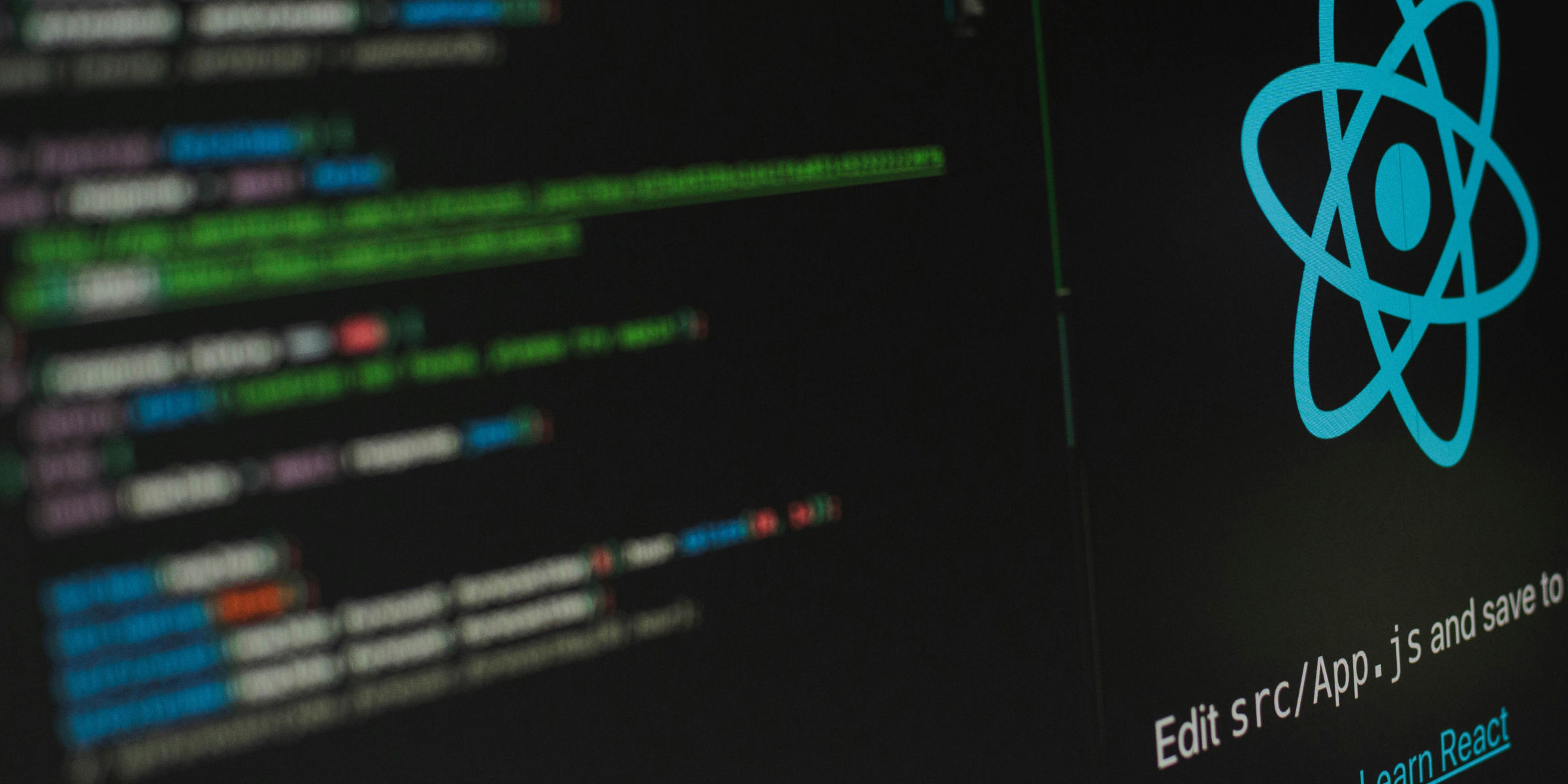
In modern web development, handling asynchronous operations is a common task, especially in React applications where data fetching and updates are frequent. Promises and Async/Await patterns are powerful tools for managing asynchronous code in JavaScript, offering cleaner and more readable solutions compared to traditional callback functions. In this blog post, we'll dive into best practices for handling asynchronous operations in React using Promises and Async/Await patterns, ensuring smooth and efficient data fetching and updates.
Understanding Promises
Promises are objects that represent the eventual completion or failure of an asynchronous operation. They provide a cleaner alternative to callback functions, allowing for better organization and error handling. Here are some key points to remember:
Key Points:
- Promises have three states: pending, fulfilled, and rejected.
- They can be chained using
.then()
and.catch()
methods. - Promises can be created using the
new Promise()
constructor or by using utility functions likefetch()
.
Using Promises in React Components
In React, Promises are commonly used for data fetching operations, such as making API calls. Here's how you can integrate Promises into your React components:
Key Points:
- Use
componentDidMount()
lifecycle method for initiating data fetching operations. - Display loading indicators while waiting for data.
- Update component state with fetched data once the Promise is resolved.
- Handle errors gracefully by catching them in a
.catch()
block.
Introducing Async/Await
Async/Await is a more modern approach to handling asynchronous code in JavaScript. It allows you to write asynchronous code in a synchronous manner, making it easier to understand and debug. Here's how it works:
Key Points:
async
functions return Promises implicitly.await
keyword pauses the execution of the function until the Promise is resolved.- Error handling can be done using
try...catch
blocks.
Implementing Async/Await in React Components
Async/Await simplifies the process of handling asynchronous operations in React components. Here's a step-by-step guide:
Key Points:
- Define an asynchronous function within your component.
- Use
await
keyword to wait for Promise resolution. - Update component state with fetched data.
- Handle errors using
try...catch
blocks for cleaner error handling.
Best Practices and Tips
To ensure smooth and efficient data fetching and updates in your React applications, consider the following best practices:
- Use Async/Await for cleaner code: Async/Await simplifies asynchronous code and makes it more readable compared to Promises and callbacks.
- Handle errors gracefully: Always implement error handling to provide a better user experience and prevent application crashes.
- Optimize data fetching: Use techniques like memoization and pagination to optimize data fetching and rendering performance.
- Keep component logic separate: Separate data fetching and rendering logic to improve code maintainability and readability.
Conclusion
Promises and Async/Await patterns are powerful tools for managing asynchronous operations in React applications. By following best practices and implementing clean and efficient code, you can ensure smooth data fetching and updates, resulting in a better user experience for your application. Happy coding
Consult us for free?
View More