Mastering Form Handling in React: Techniques and Patterns
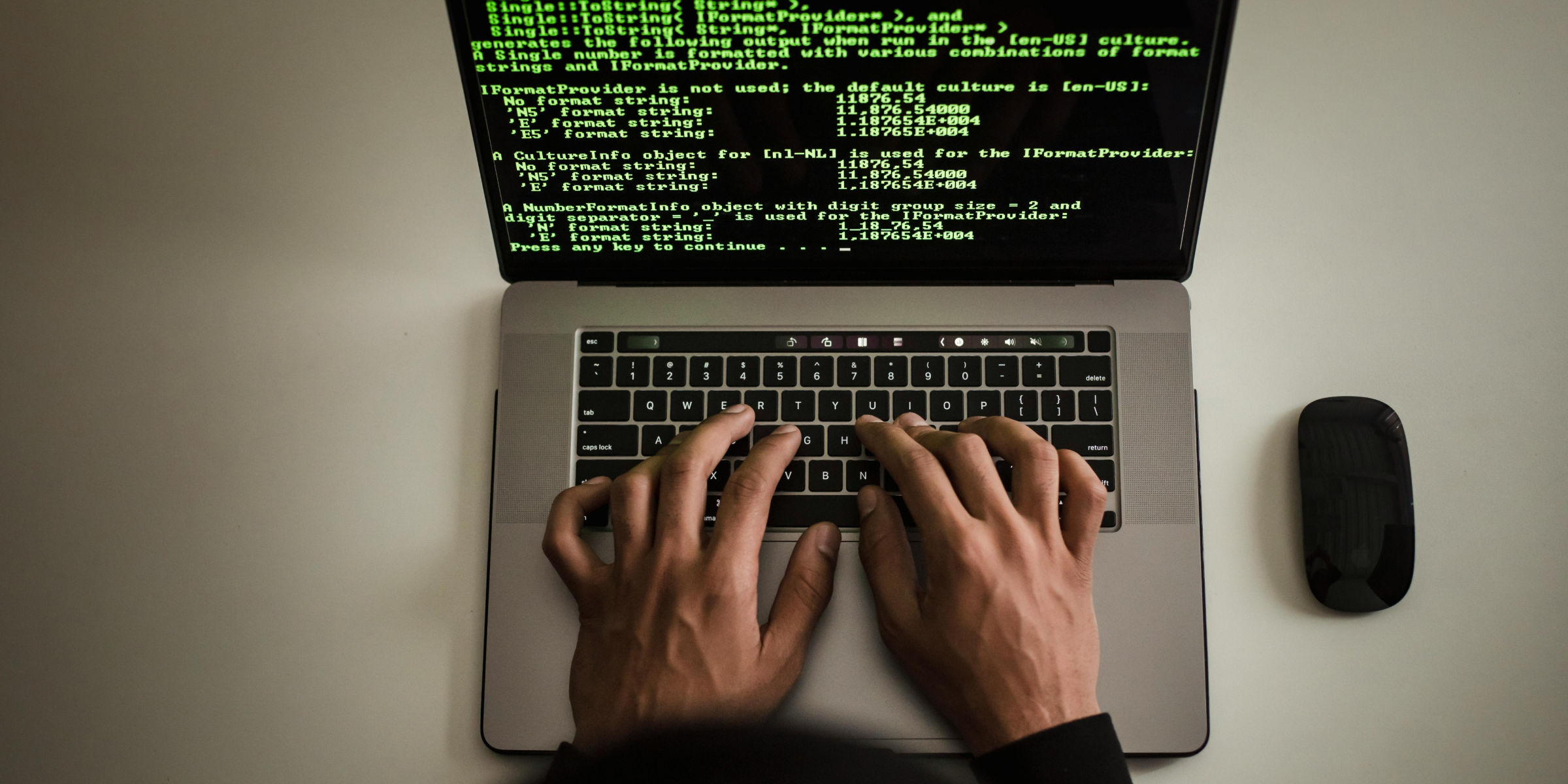
Forms are a crucial aspect of web development, and handling them effectively in React can greatly enhance user experience. In this blog, we'll delve into various techniques and patterns for mastering form handling in React applications.
Understanding Controlled Components
What are Controlled Components?
Controlled components are React components whose form elements are controlled by React state. This means that the form data is managed by React, allowing for more precise control and manipulation.
Benefits of Controlled Components
- Single Source of Truth: The form data resides in the component's state, ensuring consistency throughout the application.
- Dynamic Updates: React state updates trigger re-renders, enabling dynamic updates to form elements based on user input.
- Validation: Easily implement validation logic by leveraging React state and lifecycle methods.
Uncontrolled Components: When to Use Them
Introduction to Uncontrolled Components
Uncontrolled components are form elements whose values are not controlled by React state. Instead, they rely on the DOM to manage their state.
Use Cases for Uncontrolled Components
- Enhancing Performance: Uncontrolled components can be beneficial for performance-critical scenarios where re-rendering controlled components might cause performance issues.
- Integrating with Third-Party Libraries: Some third-party libraries or legacy code may require uncontrolled components for seamless integration.
Form Validation Strategies
Client-Side Validation
Client-side validation involves validating form data within the browser before submission. This can be achieved using various techniques such as regular expressions, custom validation functions, or third-party libraries like Yup or Formik.
Server-Side Validation
Server-side validation is essential for ensuring data integrity and security. It involves validating form data on the server-side before processing it further. React applications typically communicate with a server using APIs, allowing for server-side validation to be seamlessly integrated.
Advanced Techniques: Formik and Yup Integration
Introduction to Formik
Formik is a popular form library for React that simplifies form management by providing utilities for handling form state, validation, and submission.
Leveraging Yup for Schema Validation
Yup is a powerful schema validation library that integrates seamlessly with Formik. It allows developers to define validation schemas declaratively, making it easy to enforce complex validation rules.
Benefits of Formik and Yup Integration
- Declarative Validation: Define validation rules using simple schemas, enhancing code readability and maintainability.
- Error Handling: Formik automatically handles error messages and field states based on Yup validation schema, streamlining the validation process.
- Form Submission: Simplify form submission logic with built-in utilities provided by Formik, such as handleSubmit and handleReset.
Conclusion
Mastering form handling in React requires a solid understanding of controlled and uncontrolled components, along with effective validation strategies. By leveraging advanced techniques such as Formik and Yup integration, developers can streamline the form development process and create robust, user-friendly applications. Remember to always consider the specific requirements of your project and choose the approach that best fits your needs. Happy coding!
Consult us for free?
View More