Mastering Liskov Substitution Principle: Building Robust and Flexible Software
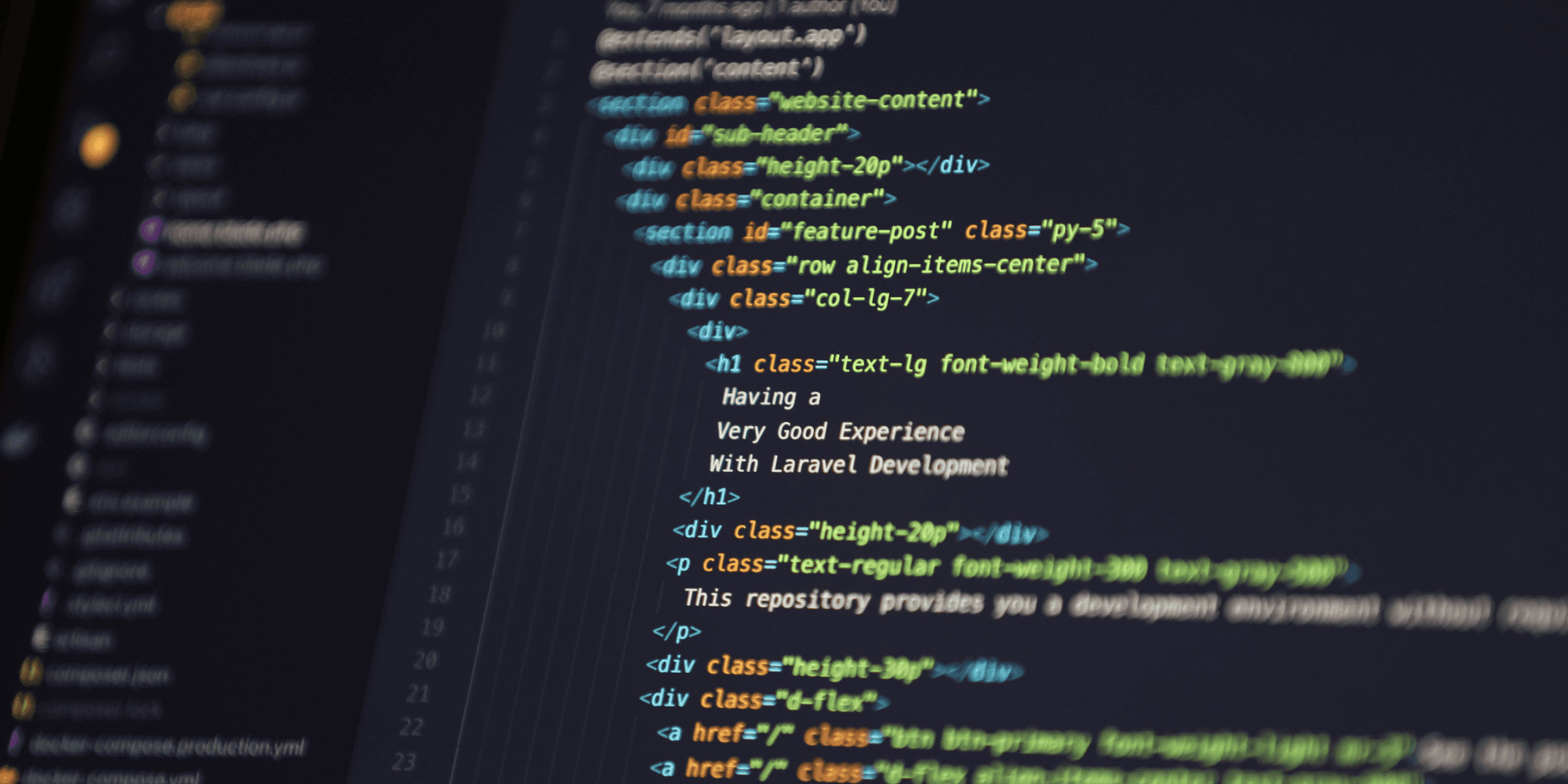
In the realm of object-oriented programming, adhering to solid principles is crucial for building software systems that are maintainable, extensible, and resilient to change. One such principle, the Liskov Substitution Principle (LSP), plays a pivotal role in achieving these goals. Named after Barbara Liskov, the principle emphasizes the importance of maintaining substitutability of objects within a class hierarchy.
Understanding Liskov Substitution Principle (LSP)
At its core, the Liskov Substitution Principle states that objects of a superclass should be replaceable with objects of its subclasses without affecting the correctness of the program. In other words, any class that inherits from a parent class should be usable in place of that parent class without altering the desirable properties of the program.
Key Tenets of LSP:
- Behavior Preservation: Subclasses should preserve the behavior of their superclass.
- No Preconditions Strengthening: Subclasses should not strengthen preconditions of the methods defined in the superclass.
- No Postconditions Weakening: Subclasses should not weaken postconditions of the methods defined in the superclass.
- Invariant Preservation: Subclasses should maintain invariants established by the superclass.
Practical Examples of LSP in Action
Let's delve into some practical examples to understand Liskov Substitution Principle better.
Example 1: Shape Hierarchy
Consider a Shape hierarchy with classes such as Rectangle, Square, and Circle. According to LSP, we should be able to substitute any subclass of Shape wherever a Shape is expected. However, violating LSP might lead to unexpected behavior. For instance, if Square inherits from Rectangle and overrides the setWidth and setHeight methods to ensure both sides are always equal, it violates LSP as it doesn't preserve the behavior of the superclass. To rectify this, we should reconsider the design and potentially refactor to ensure LSP compliance.
class Rectangle:
def set_width(self, width):
self.width = width
def set_height(self, height):
self.height = height
def area(self):
return self.width * self.height
class Square(Rectangle):
def set_width(self, width):
self.width = width
self.height = width
def set_height(self, height):
self.height = height
self.width = height
Example 2: Animal Hierarchy
Another common example is an Animal hierarchy with classes like Bird and Dog. If a method expects an Animal object, it should work seamlessly with any subclass of Animal. However, violating LSP could lead to unexpected behavior. For instance, if Bird and Dog have a method called fly() and walk() respectively, and if a method expecting an Animal object calls fly() on a Dog instance, it breaks LSP.
Benefits of Adhering to LSP
- Improved Maintainability: Code becomes easier to understand, modify, and maintain as it adheres to a consistent and predictable behavior.
- Enhanced Extensibility: New subclasses can be added to the hierarchy without impacting existing code, promoting code reuse and scalability.
- Reduced Bugs and Side Effects: By following LSP, the risk of introducing bugs and unintended side effects is minimized, resulting in more reliable software systems.
Conclusion
Mastering the Liskov Substitution Principle is essential for building robust and flexible software systems. By adhering to LSP, developers can ensure that their code is more maintainable, extensible, and resilient to change. Understanding the core concepts of LSP and applying them effectively can lead to cleaner, more reliable codebases, ultimately benefiting both developers and end-users alike.
Consult us for free?
View More