Mastering React Hooks: A Comprehensive Guide
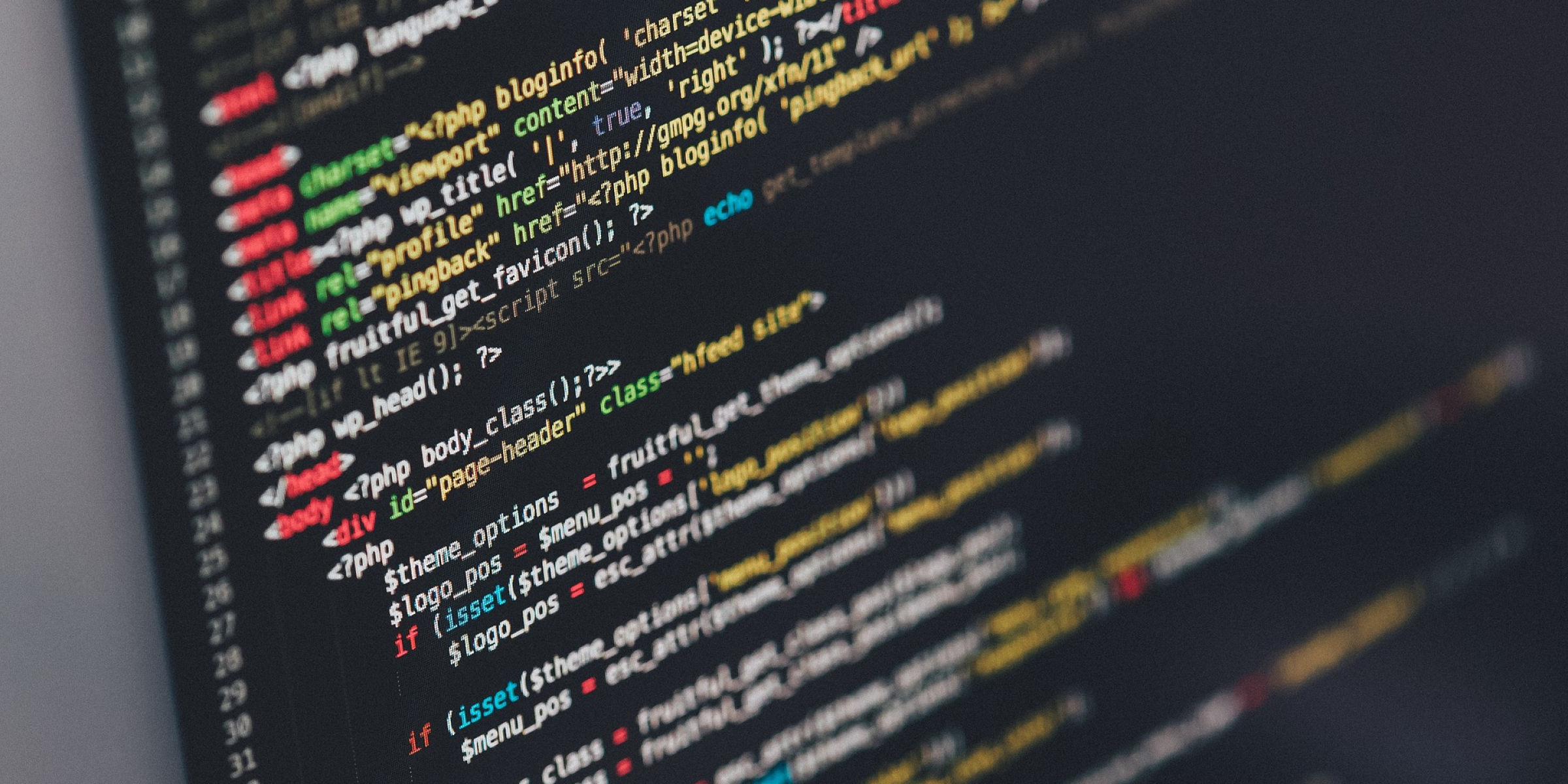
In the ever-evolving landscape of React development, React Hooks have emerged as a powerful tool for managing state and side effects in functional components. With Hooks, developers can write more concise, readable, and reusable code. In this comprehensive guide, we'll dive deep into mastering React Hooks, covering everything from the basics to advanced techniques.
Table of Contents
- Introduction to React Hooks
- Understanding the Basics of Hooks
- useState: Managing State in Functional Components
- useEffect: Handling Side Effects in Functional Components
- Custom Hooks: Reusable Logic
- Creating Custom Hooks
- Sharing Stateful Logic
- Advanced Hooks Techniques
- useContext: Simplifying Context Management
- useReducer: Managing Complex State Logic
- Best Practices and Tips
- Keep Hooks Simple and Focused
- Use Memoization for Performance Optimization
- Avoid Using Hooks Inside Loops or Conditions
- Common Patterns and Use Cases
- Form Handling with useState
- Data Fetching with useEffect
- Troubleshooting and Debugging Hooks
- Common Pitfalls and How to Avoid Them
- Using React DevTools for Hook Inspection
- Future of React Hooks
- Updates and Enhancements in React Versions
- Community Trends and Contributions
1. Introduction to React Hooks
React Hooks were introduced in React 16.8 as a way to add state and other React features to functional components. Before Hooks, stateful logic was only available in class components, leading to complex hierarchies and less reusable code. With Hooks, functional components can now manage state and side effects, making them more powerful and flexible.
2. Understanding the Basics of Hooks
useState: One of the most fundamental Hooks, useState allows functional components to have state. It returns a stateful value and a function to update it, providing a simple way to manage component state without classes.
useEffect: useEffect enables functional components to perform side effects such as data fetching, subscriptions, or manually changing the DOM. It runs after every render and can perform cleanup actions.
3. Custom Hooks: Reusable Logic
Custom Hooks allow developers to extract and reuse stateful logic across components. By creating custom Hooks, you can abstract away complex logic and make it reusable across your application.
Creating Custom Hooks: Custom Hooks are simply JavaScript functions that start with the word "use" and call other Hooks if needed. They can encapsulate any reusable piece of logic, such as form validation, data fetching, or even animations.
Sharing Stateful Logic: Custom Hooks can be shared between components, making them an excellent tool for code organization and reusability. By encapsulating logic in custom Hooks, you can create cleaner and more maintainable code.
4. Advanced Hooks Techniques
useContext: useContext provides a way to pass data through the component tree without having to pass props down manually at every level. It simplifies the process of accessing global data, such as theme preferences or authentication status.
useReducer: useReducer is an alternative to useState for managing complex state logic. It accepts a reducer function and an initial state, returning the current state and a dispatch function to update it. useReducer is particularly useful for state that involves multiple sub-values or when the next state depends on the previous one.
5. Best Practices and Tips
Keep Hooks Simple and Focused: Each Hook should ideally do one thing and do it well. Avoid combining multiple concerns into a single Hook to keep your code clean and maintainable.
Use Memoization for Performance Optimization: When using Hooks that rely on expensive computations or data fetching, memoization can help optimize performance by caching values and preventing unnecessary re-renders.
Avoid Using Hooks Inside Loops or Conditions: Hooks should always be used at the top level of functional components, not inside loops, conditions, or nested functions. This ensures that Hooks are called in the same order every time and maintain their state correctly.
6. Common Patterns and Use Cases
Form Handling with useState: useState is commonly used for form handling in React applications. By storing form values in state and updating them with onChange handlers, you can create interactive and responsive forms.
Data Fetching with useEffect: useEffect is often used for data fetching in React applications. By using useEffect to fetch data from an API and updating state with the fetched data, you can create dynamic and data-driven user interfaces.
7. Troubleshooting and Debugging Hooks
Common Pitfalls and How to Avoid Them: While Hooks offer many benefits, they also come with their own set of pitfalls and gotchas. Common issues include stale closures, infinite loops, and incorrect dependencies in useEffect. Understanding these pitfalls and how to avoid them is essential for writing bug-free code.
Using React DevTools for Hook Inspection: React DevTools provide powerful tools for inspecting and debugging Hooks in your application. You can use the "Hooks" tab to view all Hooks used by a component, their current state, and their call stack.
8. Future of React Hooks
Updates and Enhancements in React Versions: React Hooks are continually evolving, with new updates and enhancements added in each React release. Keeping up to date with the latest changes and best practices is essential for maximizing the benefits of Hooks in your applications.
Community Trends and Contributions: The React community plays a vital role in shaping the future of Hooks, with developers sharing their experiences, best practices, and custom Hooks libraries. By staying engaged with the community and contributing to open-source projects, you can help drive the evolution of Hooks and make React development more accessible and enjoyable for everyone.
Consult us for free?
View More