Simplifying Complex Logic with the Strategy Pattern
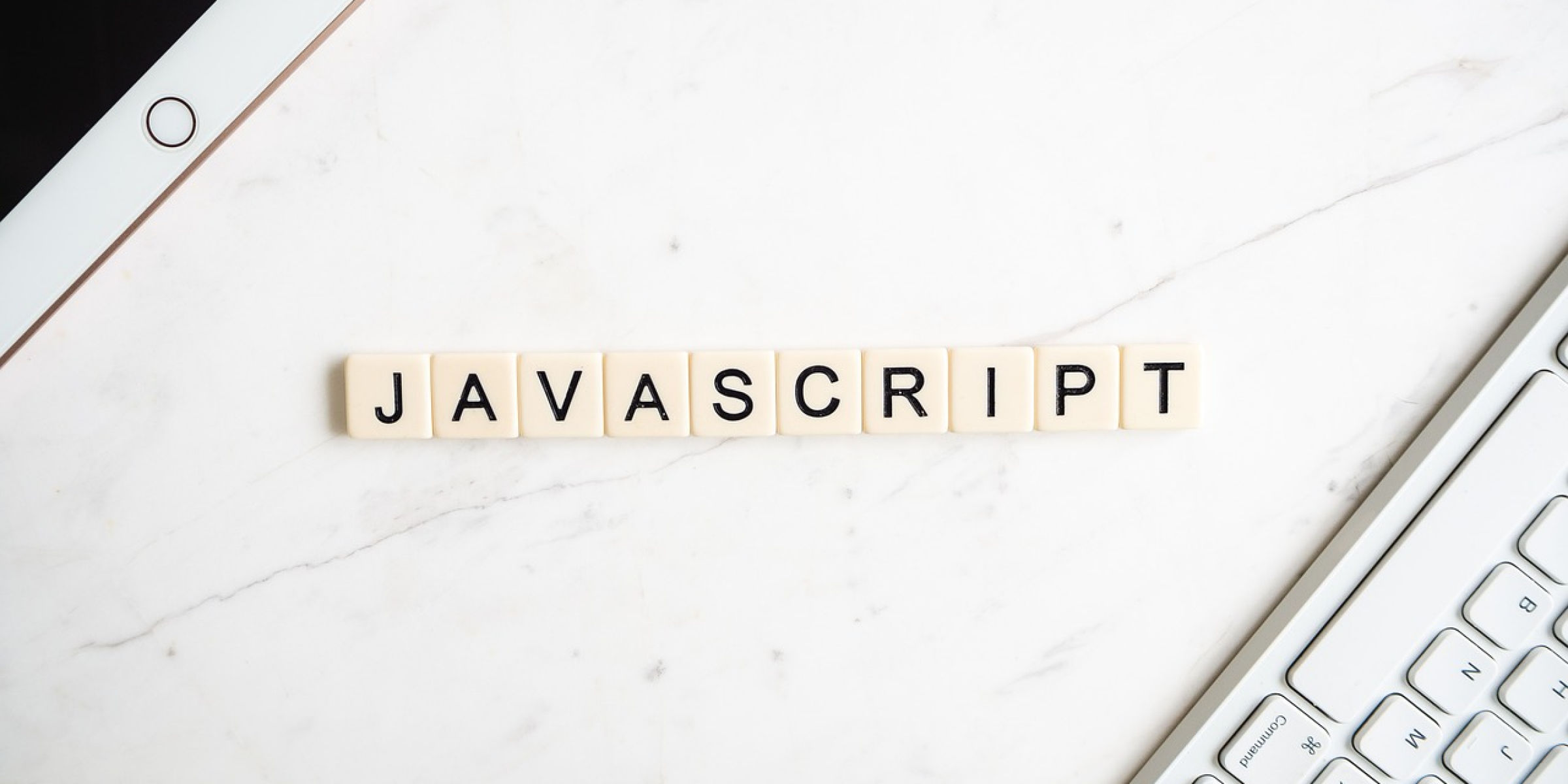
Managing complex logic in software development can become overwhelming. As requirements evolve and new features are added, maintaining and extending this logic can become a nightmare. This is where design patterns come to the rescue. One such pattern that proves invaluable in these scenarios is the Strategy pattern.
Understanding the Strategy Pattern
What is the Strategy Pattern?
At its core, the Strategy pattern is a behavioral design pattern that allows algorithms to be selected at runtime. It encapsulates these algorithms into separate classes, known as strategies, and allows the client to switch between them dynamically.
How Does it Work?
The Strategy pattern involves three main components:
- Context: This is the class that contains the complex logic and maintains a reference to the current strategy.
- Strategy Interface: An interface or abstract class that defines the common methods that all strategies must implement.
- Concrete Strategies: These are the individual algorithms encapsulated within their own classes, each implementing the strategy interface.
Applying the Strategy Pattern in JavaScript
Example Scenario: Sorting Algorithms
Let's consider a scenario where we need to sort a list of items using different sorting algorithms such as bubble sort, merge sort, and quick sort.
Implementing the Strategy Pattern
Step 1: Define the Strategy Interface
class SortStrategy {
sort(data) {
throw new Error("sort method must be implemented");
}
}
Step 2: Implement Concrete Strategies
class BubbleSort extends SortStrategy {
sort(data) {
// Implement bubble sort algorithm
return data;
}
}
class MergeSort extends SortStrategy {
sort(data) {
// Implement merge sort algorithm
return data;
}
}
class QuickSort extends SortStrategy {
sort(data) {
// Implement quick sort algorithm
return data;
}
}
Step 3: Create the Context
class Sorter {
constructor(strategy) {
this.strategy = strategy;
}
setStrategy(strategy) {
this.strategy = strategy;
}
sort(data) {
return this.strategy.sort(data);
}
}
Benefits of Using the Strategy Pattern
- Encapsulation: Each sorting algorithm is encapsulated within its own class, promoting cleaner and more maintainable code.
- Flexibility: Strategies can be easily swapped at runtime, allowing for dynamic behavior.
- Testability: Since each strategy is isolated, unit testing becomes easier and more effective.
Conclusion
The Strategy pattern is a powerful tool for simplifying complex logic in JavaScript applications. By encapsulating algorithms into separate classes and allowing dynamic switching between them, it promotes maintainability, flexibility, and testability. When faced with scenarios involving multiple algorithms or complex decision-making processes, consider leveraging the Strategy pattern to keep your codebase clean and organized.
Consult us for free?
View More