Testing Design Patterns: How to Design Testable Code in Python
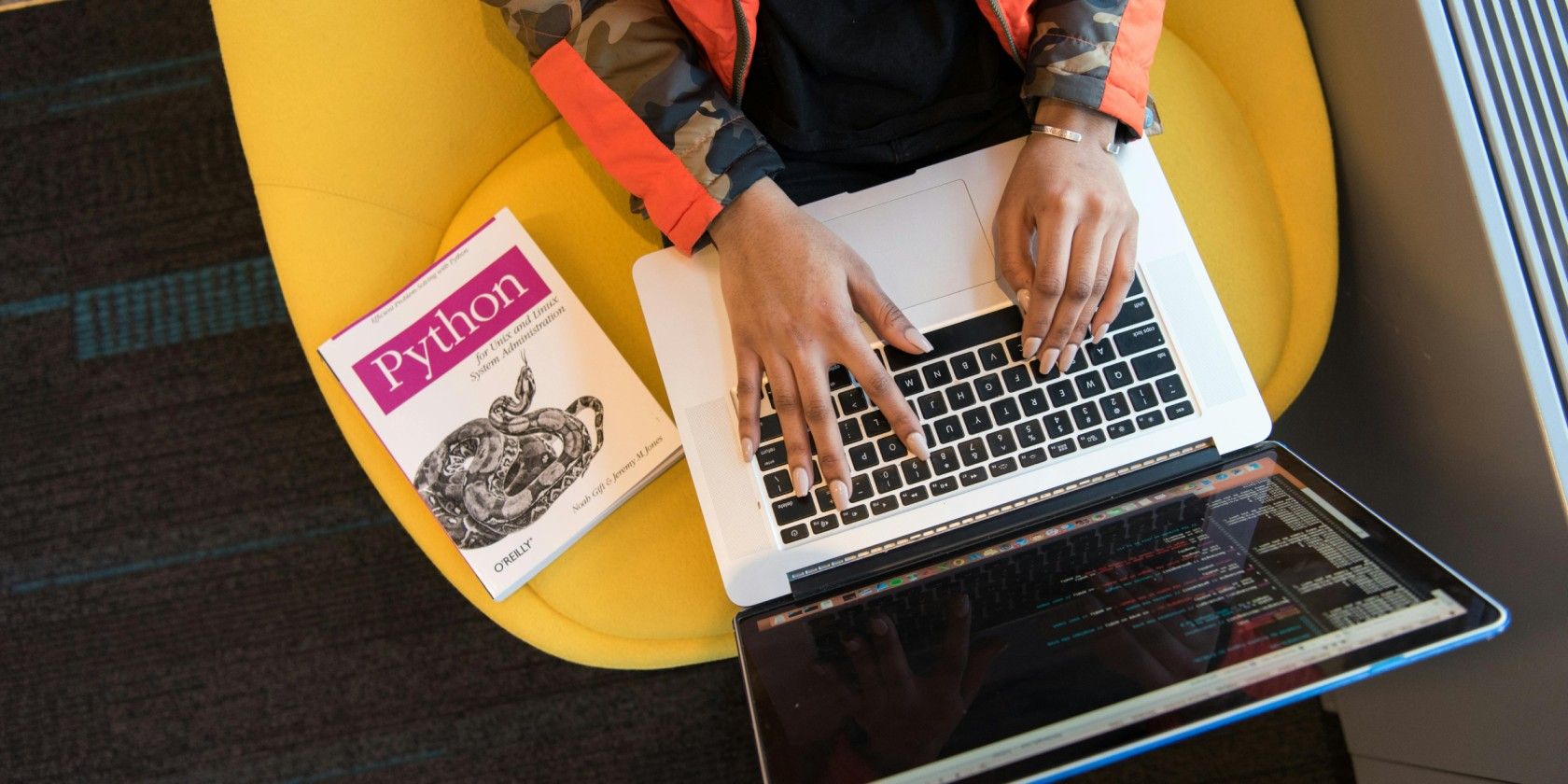
In the world of software development, writing tests is crucial to ensuring the reliability and maintainability of your codebase. However, writing effective tests can be challenging, especially when dealing with complex code structures. This is where the concept of testable code and design patterns come into play. In this blog post, we'll explore how design patterns such as Dependency Injection, Mock Objects, and Test Doubles can promote testability in Python projects, making unit testing easier and improving overall code quality.
Understanding Testable Code
What is Testability?
Testability refers to the ease with which software can be tested. A highly testable codebase allows developers to write automated tests that are reliable, maintainable, and efficient.
Why is Testability Important?
- Improved Code Quality: Testable code tends to be well-structured, modular, and easier to understand, leading to higher-quality software.
- Faster Development: With a testable codebase, developers can iterate more quickly and confidently, reducing the time spent on debugging and troubleshooting.
- Facilitates Refactoring: Testable code encourages modular design and loose coupling, making it easier to refactor and evolve the codebase over time.
Design Patterns for Testability
1. Dependency Injection
Dependency Injection (DI) is a design pattern that promotes loose coupling between components by allowing dependencies to be passed as arguments rather than hardcoding them within the component.
Key Benefits of Dependency Injection:
- Improved Testability: By injecting dependencies, components become easier to test as they can be easily replaced with mock or stub implementations during testing.
- Increased Modularity: Dependency Injection encourages modular design by explicitly defining and injecting dependencies, making components more reusable and maintainable.
- Enhanced Flexibility: Components become more flexible and configurable as dependencies can be swapped out or modified without changing the component's implementation.
2. Mock Objects
Mock Objects are dummy implementations of real objects that simulate the behavior of dependencies during testing. Mocking allows developers to isolate the code under test and verify its interactions with dependencies.
Key Benefits of Mock Objects:
- Isolation: Mock Objects enable developers to isolate the code under test by replacing real dependencies with controlled substitutes, allowing for focused unit testing.
- Behavior Verification: Mock Objects allow developers to verify the interactions between the code under test and its dependencies, ensuring that the expected interactions occur.
- Reduced Test Coupling: By using Mock Objects, tests become less coupled to the implementation details of dependencies, making tests more resilient to changes in the codebase.
3. Test Doubles
Test Doubles are generic terms used to describe objects that replace real dependencies during testing. Test Doubles include various types such as mocks, stubs, spies, and fakes, each serving a specific purpose in testing.
Key Types of Test Doubles:
- Mocks: Objects that simulate the behavior of dependencies and allow developers to verify interactions during testing.
- Stubs: Objects that provide canned responses to method calls and allow developers to control the behavior of dependencies during testing.
- Spies: Objects that record information about method calls made to dependencies, allowing developers to inspect and verify these interactions.
- Fakes: Simplified implementations of dependencies that provide functional behavior for testing purposes.
Applying Design Patterns in Python Projects
Example: Dependency Injection in Python
class EmailService:
def __init__(self, smtp_client):
self.smtp_client = smtp_client
def send_email(self, to, subject, body):
# Send email using SMTP client
self.smtp_client.send_email(to, subject, body)
class MockSMTPClient:
def send_email(self, to, subject, body):
# Simulate sending email
print(f"Email sent to {to}: {subject} - {body}")
# Injecting MockSMTPClient for testing
mock_smtp_client = MockSMTPClient()
email_service = EmailService(mock_smtp_client)
email_service.send_email("[email protected]", "Test Subject", "Test Body")
In this example, the EmailService
class depends on an SMTP client for sending emails. By injecting the SMTP client dependency, we can easily replace it with a mock implementation (MockSMTPClient
) during testing, allowing us to isolate and test the EmailService
class independently.
Conclusion
Design patterns such as Dependency Injection, Mock Objects, and Test Doubles play a crucial role in promoting testability and improving overall code quality in Python projects. By adopting these patterns, developers can design more modular, maintainable, and testable codebases, leading to faster development cycles and higher-quality software products. Remember, writing tests is not just about catching bugs but also about designing software that is resilient, flexible, and easy to maintain over time.
Consult us for free?
View More