Understanding React Router: A Complete Tutorial
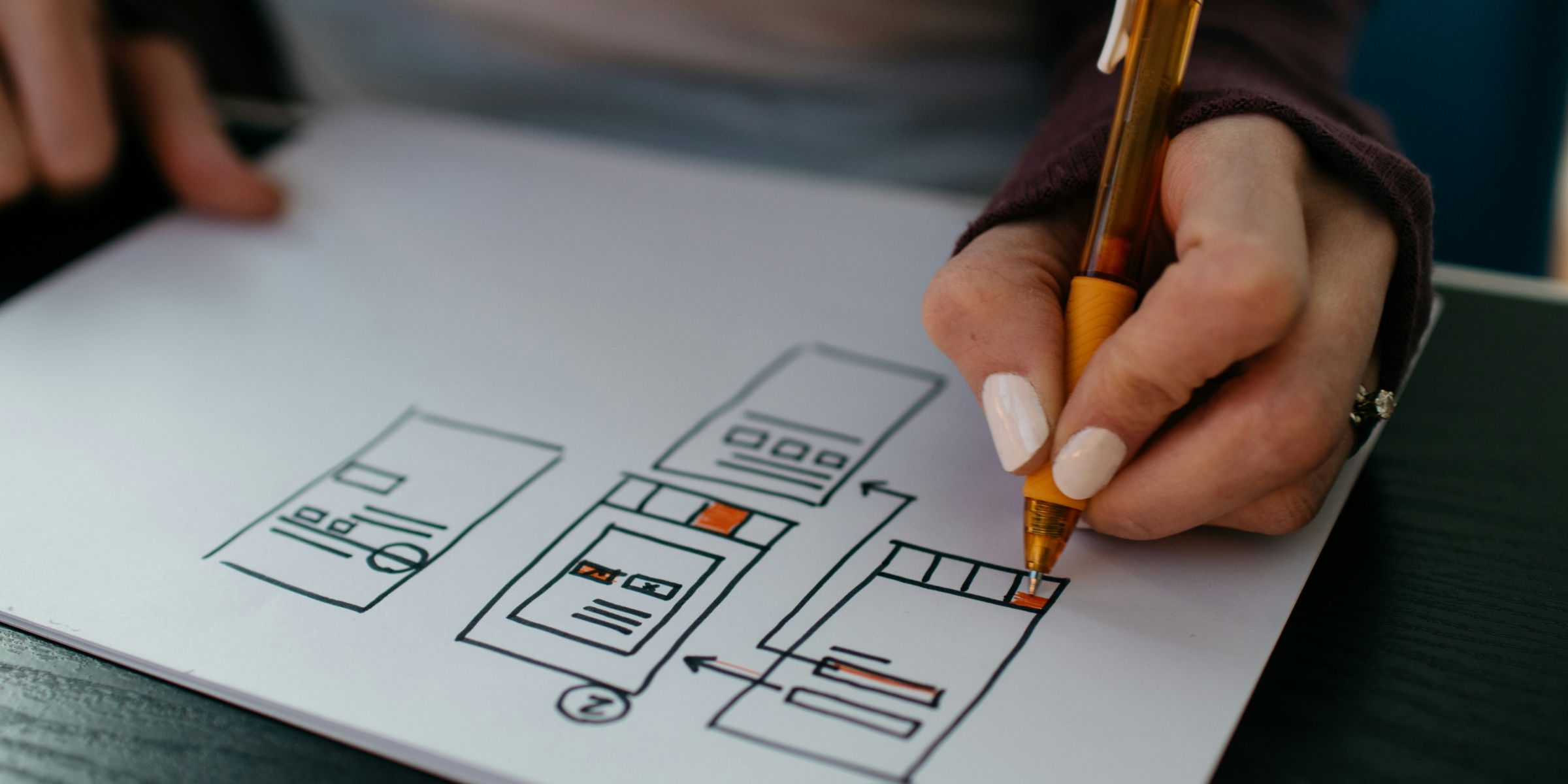
Navigating between different pages within a React application is essential for creating a smooth and intuitive user experience. React Router is a powerful library that enables developers to handle routing in their single-page applications efficiently. In this comprehensive tutorial, we will dive deep into understanding React Router and explore its various features.
What is React Router?
React Router is a routing library built specifically for React applications. It allows developers to define multiple routes in their application and render different components based on the URL path. React Router provides a declarative way to handle navigation and keeps the UI in sync with the URL.
Getting Started with React Router
Installation
To begin using React Router in your project, you can install it via npm or yarn:
npm install react-router-dom
or
yarn add react-router-dom
Basic Usage
Once installed, you can import the necessary components from react-router-dom
and start defining your routes. The BrowserRouter
component serves as the root of your application's routing configuration:
import { BrowserRouter, Route, Switch } from 'react-router-dom';
function App() {
return (
<BrowserRouter>
<Switch>
<Route path="/" exact component={Home} />
<Route path="/about" component={About} />
<Route path="/contact" component={Contact} />
<Route component={NotFound} />
</Switch>
</BrowserRouter>
);
}
Key Concepts in React Router
Route
The Route
component is used to define a mapping between a URL path and a React component. It takes two main props: path
, which specifies the URL path, and component
, which specifies the component to render when the path matches.
Switch
The Switch
component is used to render the first Route
that matches the current URL. This prevents multiple routes from being rendered simultaneously and ensures that only one component is displayed at a time.
Link
The Link
component is used to create links between different pages within the application. It renders an anchor tag (<a>
) with the specified URL path and ensures that the page does not reload when the link is clicked.
Advanced Features of React Router
Nested Routes
React Router supports nested routes, allowing you to define routes within routes. This is useful for creating complex page layouts and organizing your application's UI hierarchy.
Route Parameters
Route parameters allow you to extract dynamic segments from the URL path and pass them as props to the rendered component. This enables dynamic routing and parameterized views within your application.
Programmatic Navigation
In addition to using Link
components for navigation, React Router also provides a history
object that allows for programmatic navigation. You can use methods like push
and replace
to navigate to different pages based on user actions or application logic.
Conclusion
In this tutorial, we've covered the basics of React Router and explored its various features for handling routing in React applications. By understanding concepts such as routes, switches, links, and advanced features like nested routes and route parameters, you'll be well-equipped to build dynamic and navigable single-page applications with React Router. Happy routing!
Consult us for free?
View More